#include <Button.hpp>
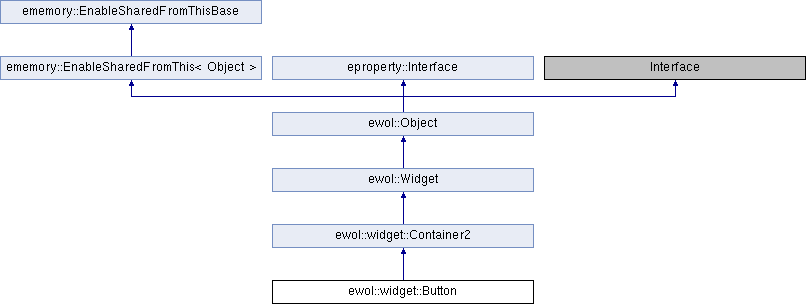
Public Types | |
enum | buttonLock { lockNone, lockWhenPressed, lockWhenReleased, lockAccess } |
Public Member Functions | |
DECLARE_WIDGET_FACTORY (Button, "Button") | |
virtual | ~Button () |
void | calculateMinMaxSize () override |
void | onChangeSize () override |
void | onRegenerateDisplay () override |
bool | onEventInput (const ewol::event::Input &_event) override |
bool | onEventEntry (const ewol::event::Entry &_event) override |
void | onDetectPresenceToggleWidget () override |
![]() | |
virtual | ~Container2 () |
void | setSubWidget (ewol::WidgetShared _subWidget) |
void | setSubWidgetToggle (ewol::WidgetShared _subWidget) |
ewol::WidgetShared | getSubWidget () const |
ewol::WidgetShared | getSubWidgetToggle () const |
void | subWidgetRemove () |
void | subWidgetRemoveToggle () |
void | subWidgetUnLink () |
void | subWidgetUnLinkToggle () |
void | systemDraw (const ewol::DrawProperty &_displayProp) override |
void | onRegenerateDisplay () override |
void | onChangeSize () override |
void | calculateMinMaxSize () override |
ewol::ObjectShared | getSubObjectNamed (const std::string &_objectName) override |
bool | loadXML (const exml::Element &_node) override |
void | setOffset (const vec2 &_newVal) override |
void | requestDestroyFromChild (const ewol::ObjectShared &_child) override |
![]() | |
virtual | ~Widget () |
virtual vec2 | relativePosition (const vec2 &_pos) |
virtual void | calculateSize () |
virtual vec2 | getSize () |
virtual void | setSize (const vec2 &_value) |
virtual vec2 | getCalculateMinSize () |
virtual vec2 | getCalculateMaxSize () |
virtual const vec2 & | getOffset () |
virtual void | setZoom (float _newVal) |
virtual float | getZoom () |
virtual void | changeZoom (float _range) |
virtual void | setOrigin (const vec2 &_pos) |
virtual vec2 | getOrigin () |
void | setNoMinSize () |
virtual void | checkMinSize () |
void | setNoMaxSize () |
virtual void | checkMaxSize () |
virtual bvec2 | canExpand () |
const bvec2 & | canFill () |
virtual bool | getFocus () |
virtual bool | setFocus () |
virtual bool | rmFocus () |
virtual void | keepFocus () |
virtual int32_t | getMouseLimit () |
virtual void | setMouseLimit (int32_t _numberState) |
virtual bool | getKeyboardRepeate () |
virtual ewol::WidgetShared | getWidgetAtPos (const vec2 &_pos) |
virtual bool | systemEventInput (ewol::event::InputSystem &_event) |
virtual bool | systemEventEntry (ewol::event::EntrySystem &_event) |
virtual void | onEventClipboard (enum gale::context::clipBoard::clipboardListe _clipboardID) |
virtual bool | onEventShortCut (const gale::key::Special &_special, char32_t _unicodeValue, enum gale::key::keyboard _kbMove, bool _isDown) |
virtual void | grabCursor () |
virtual void | unGrabCursor () |
virtual bool | getGrabStatus () |
virtual void | setCursor (enum gale::context::cursor _newCursor) |
virtual enum gale::context::cursor | getCursor () |
void | requestUpdateSize () |
ewol::widget::Manager & | getWidgetManager () |
ememory::SharedPtr< ewol::widget::Windows > | getWindows () |
void | setAnnimationType (enum ewol::Widget::annimationMode _mode, const std::string &_type) |
void | setAnnimationTime (enum ewol::Widget::annimationMode _mode, float _time) |
bool | startAnnimation (enum ewol::Widget::annimationMode _mode) |
bool | stopAnnimation () |
![]() | |
DECLARE_FACTORY (Object) | |
virtual | ~Object () |
bool | objectHasBeenCorectlyInit () |
virtual void | destroy () |
bool | isDestroyed () const |
virtual void | setParent (const ewol::ObjectShared &_newParent) |
virtual void | removeParent () |
const char *const | getObjectType () const |
std::string | getTypeDescription () const |
bool | isTypeCompatible (const std::string &_type) const |
bool | getStatic () |
int32_t | getId () |
bool | propertySetOnWidgetNamed (const std::string &_objectName, const std::string &_config, const std::string &_value) |
virtual bool | storeXML (exml::Element &_node) const |
void | setStatusResource (bool _val) |
bool | getStatusResource () const |
![]() | |
ememory::SharedPtr< EMEMORY_TYPE > | sharedFromThis () |
const ememory::SharedPtr< EMEMORY_TYPE > | sharedFromThis () const |
ememory::WeakPtr< EMEMORY_TYPE > | weakFromThis () |
const ememory::WeakPtr< EMEMORY_TYPE > | weakFromThis () const |
Protected Member Functions | |
Button () | |
void | init () override |
virtual void | onDraw () override |
void | periodicCall (const ewol::event::Time &_event) |
void | onLostFocus () override |
virtual void | onChangePropertyShape () |
virtual void | onChangePropertyValue () |
virtual void | onChangePropertyLock () |
virtual void | onChangePropertyToggleMode () |
virtual void | onChangePropertyEnableSingle () |
![]() | |
Container2 () | |
virtual ewol::Padding | onChangeSizePadded (const ewol::Padding &_padding=ewol::Padding(0, 0, 0, 0)) |
virtual void | calculateMinMaxSizePadded (const ewol::Padding &_padding=ewol::Padding(0, 0, 0, 0)) |
int32_t | convertId (int32_t _id) |
virtual void | subWidgetReplace (const ewol::WidgetShared &_oldWidget, const ewol::WidgetShared &_newWidget) |
![]() | |
Widget () | |
virtual void | onGetFocus () |
virtual void | setKeyboardRepeate (bool _state) |
virtual void | showKeyboard () |
virtual void | hideKeyboard () |
virtual void | shortCutAdd (const std::string &_descriptiveString, const std::string &_message="") |
virtual void | shortCutClean () |
virtual void | shortCutRemove (const std::string &_message) |
virtual void | markToRedraw () |
virtual bool | needRedraw () |
void | addAnnimationType (enum ewol::Widget::annimationMode _mode, const char *_type) |
virtual bool | onStartAnnimation (enum ewol::Widget::annimationMode _mode) |
virtual void | onStopAnnimation () |
virtual void | onChangePropertyCanFocus () |
virtual void | onChangePropertyGravity () |
virtual void | onChangePropertyHide () |
virtual void | onChangePropertyFill () |
virtual void | onChangePropertyExpand () |
virtual void | onChangePropertyMaxSize () |
virtual void | onChangePropertyMinSize () |
![]() | |
Object () | |
virtual void | autoDestroy () |
void | addObjectType (const char *_type) |
Protected Attributes | |
esignal::Connection | m_PCH |
![]() | |
ewol::WidgetShared | m_subWidget [2] |
int32_t | m_idWidgetDisplayed |
![]() | |
vec2 | m_size |
vec2 | m_minSize |
vec2 | m_maxSize |
vec2 | m_offset |
float | m_zoom |
vec2 | m_origin |
bool | m_needRegenerateDisplay |
enum annimationMode | m_annimationMode |
float | m_annimationratio |
eproperty::List< int32_t > | propertyAnnimationTypeStart |
eproperty::Range< float > | propertyAnnimationTimeStart |
eproperty::List< int32_t > | propertyAnnimationTypeStop |
eproperty::Range< float > | propertyAnnimationTimeStop |
![]() | |
ewol::ObjectWeak | m_parent |
bool | m_destroy |
bool | m_static |
Additional Inherited Members | |
![]() | |
static ewol::object::Manager & | getObjectManager () |
static ewol::Context & | getContext () |
static ewol::ObjectShared | getObjectNamed (const std::string &_objectName) |
![]() | |
enum | annimationMode { annimationModeEnableAdd, annimationModeEnableRemove, annimationModeDisable } |
Detailed Description
a composed button is a button with an inside composed with the specify XML element ==> this permit to generate standard element simple
Member Enumeration Documentation
§ buttonLock
Constructor & Destructor Documentation
§ Button()
|
protected |
Constructor.
- Parameters
-
[in] _shaperName Shaper file properties
§ ~Button()
|
virtual |
Destructor.
Member Function Documentation
§ calculateMinMaxSize()
|
overridevirtual |
calculate the minimum and maximum size (need to estimate expend properties of the widget)
- Note
- : INTERNAL EWOL SYSTEM
Reimplemented from ewol::Widget.
§ onChangeSize()
|
overridevirtual |
Parent have set the size and the origin. the container need to update the subwidget property.
- Note
- : INTERNAL EWOL SYSTEM
Reimplemented from ewol::Widget.
§ onDetectPresenceToggleWidget()
|
inlineoverridevirtual |
Called when parsing a XML and detect the presence of a second Widget.
Reimplemented from ewol::widget::Container2.
§ onDraw()
|
overrideprotectedvirtual |
Common widget drawing function (called by the drawing thread [Android, X11, ...])
Reimplemented from ewol::Widget.
§ onEventEntry()
|
overridevirtual |
Entry event. represent the physical event :
- Keyboard (key event and move event)
- Accelerometer
- Joystick
- Parameters
-
[in] _event Event properties
- Returns
- true if the event has been used
- false if the event has not been used
Reimplemented from ewol::Widget.
§ onEventInput()
|
overridevirtual |
Event on an input of this Widget (finger, mouse, stilet)
- Parameters
-
[in] _event Event properties
- Returns
- true the event is used
- false the event is not used
Reimplemented from ewol::Widget.
§ onLostFocus()
|
overrideprotectedvirtual |
Event of the focus has been lost by the current widget.
Reimplemented from ewol::Widget.
§ onRegenerateDisplay()
|
overridevirtual |
Event generated when a redraw is needed.
Reimplemented from ewol::Widget.
§ periodicCall()
|
protected |
Periodic call to update grapgic display.
- Parameters
-
[in] _event Time generic event
Member Data Documentation
§ m_PCH
|
protected |
Periodic Call Handle to remove it when needed.
§ propertyEnableSingle
eproperty::Value<bool> ewol::widget::Button::propertyEnableSingle |
When a single subwidget is set display all time it.
§ propertyLock
eproperty::List<enum buttonLock> ewol::widget::Button::propertyLock |
Current lock state of the button.
§ propertyShape
eproperty::Value<std::string> ewol::widget::Button::propertyShape |
shaper name property
§ propertyToggleMode
eproperty::Value<bool> ewol::widget::Button::propertyToggleMode |
The button is able to toggle.
§ propertyValue
eproperty::Value<bool> ewol::widget::Button::propertyValue |
Current state of the button.
The documentation for this class was generated from the following file:
- framework/atria-soft/ewol/ewol/widget/Button.hpp