#include <Object.hpp>
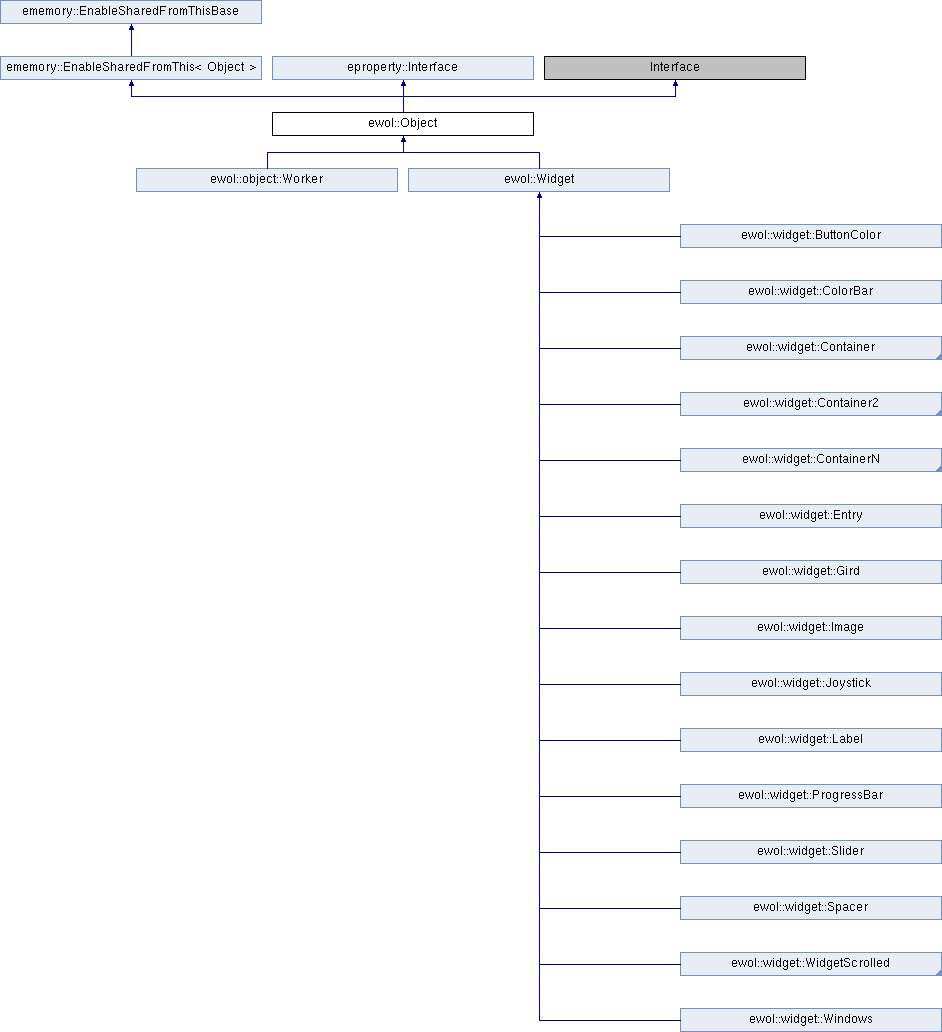
Public Member Functions | |
DECLARE_FACTORY (Object) | |
virtual | ~Object () |
bool | objectHasBeenCorectlyInit () |
virtual void | destroy () |
bool | isDestroyed () const |
virtual void | setParent (const ewol::ObjectShared &_newParent) |
virtual void | removeParent () |
const char *const | getObjectType () const |
std::string | getTypeDescription () const |
bool | isTypeCompatible (const std::string &_type) const |
bool | getStatic () |
int32_t | getId () |
bool | propertySetOnWidgetNamed (const std::string &_objectName, const std::string &_config, const std::string &_value) |
virtual bool | loadXML (const exml::Element &_node) |
virtual bool | storeXML (exml::Element &_node) const |
void | setStatusResource (bool _val) |
bool | getStatusResource () const |
virtual ewol::ObjectShared | getSubObjectNamed (const std::string &_objectName) |
![]() | |
ememory::SharedPtr< EMEMORY_TYPE > | sharedFromThis () |
const ememory::SharedPtr< EMEMORY_TYPE > | sharedFromThis () const |
ememory::WeakPtr< EMEMORY_TYPE > | weakFromThis () |
const ememory::WeakPtr< EMEMORY_TYPE > | weakFromThis () const |
Static Public Member Functions | |
static ewol::object::Manager & | getObjectManager () |
static ewol::Context & | getContext () |
static ewol::ObjectShared | getObjectNamed (const std::string &_objectName) |
Public Attributes | |
eproperty::Value< std::string > | propertyName |
![]() | |
eproperty::InterfaceData | properties |
Protected Member Functions | |
Object () | |
virtual void | init () |
virtual void | autoDestroy () |
virtual void | requestDestroyFromChild (const ewol::ObjectShared &_child) |
void | addObjectType (const char *_type) |
Protected Attributes | |
ewol::ObjectWeak | m_parent |
bool | m_destroy |
bool | m_static |
Detailed Description
Basic message classes for ewol system this class mermit at every Object to communicate between them.
Constructor & Destructor Documentation
§ Object()
|
protected |
Constructor.
§ ~Object()
|
virtual |
Destructor.
Member Function Documentation
§ addObjectType()
|
protected |
Add a type of the list of Object.
- Parameters
-
[in] _type new type to add.
§ autoDestroy()
|
protectedvirtual |
Auto-destroy the object.
§ DECLARE_FACTORY()
ewol::Object::DECLARE_FACTORY | ( | Object | ) |
Factory.
§ destroy()
|
virtual |
Destroy the current object.
Reimplemented in ewol::object::Worker.
§ getContext()
|
static |
get the curent the system inteface.
- Returns
- current reference on the instance.
§ getId()
|
inline |
get the UniqueId of the Object
- Returns
- the requested ID
§ getObjectManager()
|
static |
get the current Object manager.
- Returns
- the requested object manager.
§ getObjectNamed()
|
static |
Retrive an object with his name (in the global list)
- Parameters
-
[in] _name Name of the object
- Returns
- the requested object or nullptr
§ getObjectType()
const char* const ewol::Object::getObjectType | ( | ) | const |
§ getStatic()
|
inline |
get the static status of the Object == > mark at true if the user set the object mark as static allocated element ==> not auto remove element
- Returns
- true if it might not be removed == > usefull for conficuration class
§ getStatusResource()
|
inline |
Get the resource status of the element.
- Returns
- the resource status.
§ getSubObjectNamed()
|
virtual |
Retrive an object with his name (in the global list)
- Parameters
-
[in] _name Name of the object
- Returns
- the requested object or nullptr
Reimplemented in ewol::widget::Container2, ewol::widget::ContainerN, ewol::widget::Windows, and ewol::widget::Container.
§ getTypeDescription()
std::string ewol::Object::getTypeDescription | ( | ) | const |
Get the herarchie of the Object type.
- Returns
- descriptive string.
§ isDestroyed()
bool ewol::Object::isDestroyed | ( | ) | const |
Check if the current objetc his destroy (in removing)
- Returns
- true The object is removed
- false The object is not removed
§ isTypeCompatible()
bool ewol::Object::isTypeCompatible | ( | const std::string & | _type | ) | const |
check if the element herited from a specific type
- Parameters
-
[in] _type Type to check.
- Returns
- true if the element is compatible.
§ loadXML()
|
virtual |
load properties with an XML node.
- Parameters
-
[in] _node Pointer on the tinyXML node.
- Returns
- true : All has been done corectly.
- false : An error occured.
Reimplemented in ewol::Widget, ewol::widget::Container2, ewol::widget::ContainerN, ewol::widget::SpinBase, ewol::widget::Container, ewol::widget::Image, ewol::widget::Label, and ewol::widget::Select.
§ removeParent()
|
virtual |
Remove the current parenting.
§ requestDestroyFromChild()
|
protectedvirtual |
Called by a whild that want to remove pointer of itself from the current list of his parrent.
- Parameters
-
[in] _child Object of the child that want to remove itself
Reimplemented in ewol::widget::Container2, ewol::widget::ContainerN, ewol::widget::Windows, and ewol::widget::Container.
§ setParent()
|
virtual |
§ setStatusResource()
|
inline |
Declare this element as a resource (or singleton) this mean the element will not be auto Remove at the end of the programm. It just notify that it is not removed.
- Parameters
-
[in] _val Value of the type of the element.
§ storeXML()
|
virtual |
store properties in this XML node.
- Parameters
-
[in,out] _node Pointer on the tinyXML node.
- Returns
- true : All has been done corectly.
- false : An error occured.
Member Data Documentation
§ m_destroy
|
protected |
Flag to know if the object is requesting has destroy.
§ m_parent
|
protected |
Reference on the current parrent.
§ m_static
|
protected |
set this variable at true if this element must not be auto destroy (exemple : use static object)
§ propertyName
eproperty::Value<std::string> ewol::Object::propertyName |
name of the element ...
The documentation for this class was generated from the following file:
- framework/atria-soft/ewol/ewol/object/Object.hpp