#include <Container2.hpp>
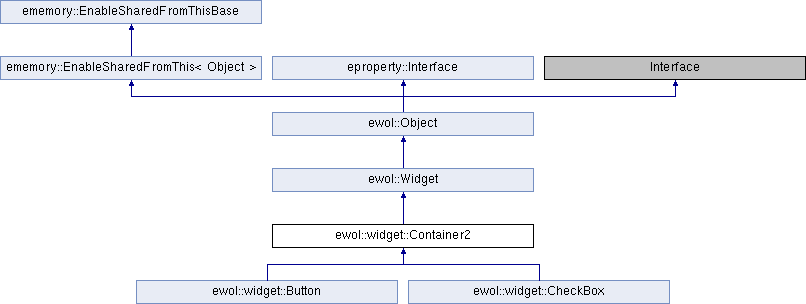
Public Member Functions | |
virtual | ~Container2 () |
void | setSubWidget (ewol::WidgetShared _subWidget) |
void | setSubWidgetToggle (ewol::WidgetShared _subWidget) |
ewol::WidgetShared | getSubWidget () const |
ewol::WidgetShared | getSubWidgetToggle () const |
void | subWidgetRemove () |
void | subWidgetRemoveToggle () |
void | subWidgetUnLink () |
void | subWidgetUnLinkToggle () |
void | systemDraw (const ewol::DrawProperty &_displayProp) override |
void | onRegenerateDisplay () override |
void | onChangeSize () override |
void | calculateMinMaxSize () override |
ewol::ObjectShared | getSubObjectNamed (const std::string &_objectName) override |
bool | loadXML (const exml::Element &_node) override |
void | setOffset (const vec2 &_newVal) override |
void | requestDestroyFromChild (const ewol::ObjectShared &_child) override |
![]() | |
virtual | ~Widget () |
virtual vec2 | relativePosition (const vec2 &_pos) |
virtual void | calculateSize () |
virtual vec2 | getSize () |
virtual void | setSize (const vec2 &_value) |
virtual vec2 | getCalculateMinSize () |
virtual vec2 | getCalculateMaxSize () |
virtual const vec2 & | getOffset () |
virtual void | setZoom (float _newVal) |
virtual float | getZoom () |
virtual void | changeZoom (float _range) |
virtual void | setOrigin (const vec2 &_pos) |
virtual vec2 | getOrigin () |
void | setNoMinSize () |
virtual void | checkMinSize () |
void | setNoMaxSize () |
virtual void | checkMaxSize () |
virtual bvec2 | canExpand () |
const bvec2 & | canFill () |
virtual bool | getFocus () |
virtual bool | setFocus () |
virtual bool | rmFocus () |
virtual void | keepFocus () |
virtual int32_t | getMouseLimit () |
virtual void | setMouseLimit (int32_t _numberState) |
virtual bool | getKeyboardRepeate () |
virtual ewol::WidgetShared | getWidgetAtPos (const vec2 &_pos) |
virtual bool | systemEventInput (ewol::event::InputSystem &_event) |
virtual bool | systemEventEntry (ewol::event::EntrySystem &_event) |
virtual void | onEventClipboard (enum gale::context::clipBoard::clipboardListe _clipboardID) |
virtual bool | onEventShortCut (const gale::key::Special &_special, char32_t _unicodeValue, enum gale::key::keyboard _kbMove, bool _isDown) |
virtual void | grabCursor () |
virtual void | unGrabCursor () |
virtual bool | getGrabStatus () |
virtual void | setCursor (enum gale::context::cursor _newCursor) |
virtual enum gale::context::cursor | getCursor () |
void | requestUpdateSize () |
ewol::widget::Manager & | getWidgetManager () |
ememory::SharedPtr< ewol::widget::Windows > | getWindows () |
void | setAnnimationType (enum ewol::Widget::annimationMode _mode, const std::string &_type) |
void | setAnnimationTime (enum ewol::Widget::annimationMode _mode, float _time) |
bool | startAnnimation (enum ewol::Widget::annimationMode _mode) |
bool | stopAnnimation () |
![]() | |
DECLARE_FACTORY (Object) | |
virtual | ~Object () |
bool | objectHasBeenCorectlyInit () |
virtual void | destroy () |
bool | isDestroyed () const |
virtual void | setParent (const ewol::ObjectShared &_newParent) |
virtual void | removeParent () |
const char *const | getObjectType () const |
std::string | getTypeDescription () const |
bool | isTypeCompatible (const std::string &_type) const |
bool | getStatic () |
int32_t | getId () |
bool | propertySetOnWidgetNamed (const std::string &_objectName, const std::string &_config, const std::string &_value) |
virtual bool | storeXML (exml::Element &_node) const |
void | setStatusResource (bool _val) |
bool | getStatusResource () const |
![]() | |
ememory::SharedPtr< EMEMORY_TYPE > | sharedFromThis () |
const ememory::SharedPtr< EMEMORY_TYPE > | sharedFromThis () const |
ememory::WeakPtr< EMEMORY_TYPE > | weakFromThis () |
const ememory::WeakPtr< EMEMORY_TYPE > | weakFromThis () const |
Protected Member Functions | |
Container2 () | |
virtual ewol::Padding | onChangeSizePadded (const ewol::Padding &_padding=ewol::Padding(0, 0, 0, 0)) |
virtual void | calculateMinMaxSizePadded (const ewol::Padding &_padding=ewol::Padding(0, 0, 0, 0)) |
virtual void | onDetectPresenceToggleWidget () |
int32_t | convertId (int32_t _id) |
virtual void | subWidgetReplace (const ewol::WidgetShared &_oldWidget, const ewol::WidgetShared &_newWidget) |
![]() | |
Widget () | |
virtual void | onGetFocus () |
virtual void | onLostFocus () |
virtual void | setKeyboardRepeate (bool _state) |
virtual void | showKeyboard () |
virtual void | hideKeyboard () |
virtual bool | onEventInput (const ewol::event::Input &_event) |
virtual bool | onEventEntry (const ewol::event::Entry &_event) |
virtual void | shortCutAdd (const std::string &_descriptiveString, const std::string &_message="") |
virtual void | shortCutClean () |
virtual void | shortCutRemove (const std::string &_message) |
virtual void | markToRedraw () |
virtual bool | needRedraw () |
virtual void | onDraw () |
void | addAnnimationType (enum ewol::Widget::annimationMode _mode, const char *_type) |
virtual bool | onStartAnnimation (enum ewol::Widget::annimationMode _mode) |
virtual void | onStopAnnimation () |
virtual void | onChangePropertyCanFocus () |
virtual void | onChangePropertyGravity () |
virtual void | onChangePropertyHide () |
virtual void | onChangePropertyFill () |
virtual void | onChangePropertyExpand () |
virtual void | onChangePropertyMaxSize () |
virtual void | onChangePropertyMinSize () |
![]() | |
Object () | |
virtual void | init () |
virtual void | autoDestroy () |
void | addObjectType (const char *_type) |
Protected Attributes | |
ewol::WidgetShared | m_subWidget [2] |
int32_t | m_idWidgetDisplayed |
![]() | |
vec2 | m_size |
vec2 | m_minSize |
vec2 | m_maxSize |
vec2 | m_offset |
float | m_zoom |
vec2 | m_origin |
bool | m_needRegenerateDisplay |
enum annimationMode | m_annimationMode |
float | m_annimationratio |
eproperty::List< int32_t > | propertyAnnimationTypeStart |
eproperty::Range< float > | propertyAnnimationTimeStart |
eproperty::List< int32_t > | propertyAnnimationTypeStop |
eproperty::Range< float > | propertyAnnimationTimeStop |
![]() | |
ewol::ObjectWeak | m_parent |
bool | m_destroy |
bool | m_static |
Additional Inherited Members | |
![]() | |
static ewol::object::Manager & | getObjectManager () |
static ewol::Context & | getContext () |
static ewol::ObjectShared | getObjectNamed (const std::string &_objectName) |
![]() | |
eproperty::Value< gale::Dimension > | propertyMinSize |
eproperty::Value< gale::Dimension > | propertyMaxSize |
eproperty::Value< bvec2 > | propertyExpand |
eproperty::Value< bvec2 > | propertyFill |
eproperty::Value< bool > | propertyHide |
eproperty::List< enum ewol::gravity > | propertyGravity |
eproperty::Value< bool > | propertyCanFocus |
esignal::Signal< std::string > | signalShortcut |
esignal::Signal | signalAnnimationStart |
esignal::Signal< float > | signalAnnimationRatio |
esignal::Signal | signalAnnimationStop |
![]() | |
eproperty::Value< std::string > | propertyName |
![]() | |
eproperty::InterfaceData | properties |
![]() | |
enum | annimationMode { annimationModeEnableAdd, annimationModeEnableRemove, annimationModeDisable } |
Detailed Description
the Cotainer widget is a widget that have an only one subWidget
Constructor & Destructor Documentation
§ Container2()
|
protected |
§ ~Container2()
|
virtual |
Destructor.
Member Function Documentation
§ calculateMinMaxSize()
|
inlineoverridevirtual |
calculate the minimum and maximum size (need to estimate expend properties of the widget)
- Note
- : INTERNAL EWOL SYSTEM
Reimplemented from ewol::Widget.
§ calculateMinMaxSizePadded()
|
protectedvirtual |
calculate the minimum and maximum size (need to estimate expend properties of the widget)
- Parameters
-
[in] _padding Padding of the widget.
- Note
- : INTERNAL EWOL SYSTEM
§ convertId()
|
inlineprotected |
convert ID of the widget if not existed
- Parameters
-
[in] _id Id of the widget to display.
- Returns
- the id of the widget displayable
§ getSubObjectNamed()
|
overridevirtual |
Retrive an object with his name (in the global list)
- Parameters
-
[in] _name Name of the object
- Returns
- the requested object or nullptr
Reimplemented from ewol::Object.
§ getSubWidget()
|
inline |
get the current displayed composition
- Returns
- The base widget
§ getSubWidgetToggle()
|
inline |
get the current displayed composition
- Returns
- The toggle widget
§ loadXML()
|
overridevirtual |
load properties with an XML node.
- Parameters
-
[in] _node Pointer on the tinyXML node.
- Returns
- true : All has been done corectly.
- false : An error occured.
Reimplemented from ewol::Widget.
§ onChangeSize()
|
inlineoverridevirtual |
Parent have set the size and the origin. the container need to update the subwidget property.
- Note
- : INTERNAL EWOL SYSTEM
Reimplemented from ewol::Widget.
§ onChangeSizePadded()
|
protectedvirtual |
Parent set the possible diplay size of the current widget whith his own possibilities By default this save the widget available size in the widget size.
- Parameters
-
[in] _padding Padding of the widget.
- Note
- : INTERNAL EWOL SYSTEM
§ onDetectPresenceToggleWidget()
|
inlineprotectedvirtual |
Called when parsing a XML and detect the presence of a second Widget.
Reimplemented in ewol::widget::Button.
§ onRegenerateDisplay()
|
overridevirtual |
Event generated when a redraw is needed.
Reimplemented from ewol::Widget.
§ requestDestroyFromChild()
|
overridevirtual |
Called by a whild that want to remove pointer of itself from the current list of his parrent.
- Parameters
-
[in] _child Object of the child that want to remove itself
Reimplemented from ewol::Object.
§ setOffset()
|
overridevirtual |
set the zoom property of the widget.
- Parameters
-
[in] _newVal offset value.
Reimplemented from ewol::Widget.
§ setSubWidget()
|
inline |
Specify the current widget.
- Parameters
-
[in] _subWidget Widget to add normal
§ setSubWidgetToggle()
|
inline |
Specify the current toggle widget.
- Parameters
-
[in] _subWidget Widget to add Toggle
§ subWidgetRemove()
|
inline |
remove the subWidget node (async).
§ subWidgetRemoveToggle()
|
inline |
remove the subWidget Toggle node (async).
§ subWidgetReplace()
|
protectedvirtual |
Replace a old subwidget with a new one.
- Parameters
-
[in] _oldWidget The widget to replace. [in] _newWidget The widget to set.
§ subWidgetUnLink()
|
inline |
Unlink the subwidget Node.
§ subWidgetUnLinkToggle()
|
inline |
Unlink the subwidget Toggle Node.
§ systemDraw()
|
overridevirtual |
{SYSTEM} extern interface to request a draw ... (called by the drawing thread [Android, X11, ...]) This function generate a clipping with the viewport openGL system. Like this a widget draw can not draw over an other widget
- Note
- This function is virtual for the scrolled widget, and the more complicated openGl widget
- Parameters
-
[in] _displayProp properties of the current display
- Note
- : INTERNAL EWOL SYSTEM
Reimplemented from ewol::Widget.
Member Data Documentation
§ m_idWidgetDisplayed
|
protected |
current widget displayed
§ m_subWidget
|
protected |
2 subwidget possible
The documentation for this class was generated from the following file:
- framework/atria-soft/ewol/ewol/widget/Container2.hpp