#include <List.hpp>
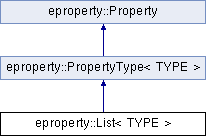
Public Member Functions | |
template<class CLASS_TYPE > | |
List (CLASS_TYPE *_owner, const std::string &_name, const TYPE &_defaultValue, const std::string &_description="", void(CLASS_TYPE::*_setObs)()=nullptr) | |
List (const TYPE &_defaultValue) | |
virtual | ~List ()=default |
void | add (const TYPE &_value, const std::string &_name, const std::string &_description="") |
void | remove (const std::string &_name) |
void | rename (const std::string &_nameOld, const std::string &_nameNew) |
std::string | getPropertyType () const override |
void | setString (const std::string &_newVal) override |
std::string | getInfo () const override |
std::vector< std::string > | getListValue () const override |
void | set (const TYPE &_newVal) override |
void | setDirectCheck (const TYPE &_newVal) override |
![]() | |
template<class CLASS_TYPE > | |
PropertyType (CLASS_TYPE *_owner, const std::string &_name, const TYPE &_defaultValue, const std::string &_description="", void(CLASS_TYPE::*_setObs)()=nullptr) | |
PropertyType (const TYPE &_defaultValue) | |
virtual | ~PropertyType ()=default |
std::string | getPropertyType () const override |
std::string | getType () const override |
std::string | getString () const override |
std::string | getDefault () const override |
std::string | getInfo () const override |
bool | isDefault () const override |
void | setDefault () override |
virtual void | changeDefault (const TYPE &_newDefault) |
const TYPE & | get () const |
void | setDirect (const TYPE &_newVal) |
TYPE & | getDirect () |
operator const TYPE & () const | |
const TYPE & | operator* () const noexcept |
const TYPE * | operator-> () const noexcept |
PropertyType< TYPE > & | operator= (const TYPE &_newVal)=delete |
![]() | |
Property (eproperty::Interface *_paramInterfaceLink, const std::string &_name) | |
Property () | |
virtual | ~Property ()=default |
void | notifyChange () const |
virtual std::string | getName () const |
template<class TYPE > | |
bool | operator== (const TYPE &_obj) const =delete |
template<class TYPE > | |
bool | operator!= (const TYPE &_obj) const =delete |
template<class TYPE > | |
bool | operator<= (const TYPE &_obj) const =delete |
template<class TYPE > | |
bool | operator>= (const TYPE &_obj) const =delete |
template<class TYPE > | |
bool | operator< (const TYPE &_obj) const =delete |
template<class TYPE > | |
bool | operator> (const TYPE &_obj) const =delete |
Additional Inherited Members | |
![]() | |
using | Observer = std::function< void()> |
![]() | |
void | setObserver (eproperty::Property::Observer _setObs) |
![]() | |
TYPE | m_value |
TYPE | m_default |
Detailed Description
template<class TYPE>
class eproperty::List< TYPE >
Set a list of value availlable (for enumeration)
Constructor & Destructor Documentation
§ List() [1/2]
|
inline |
Create a parameter with List of element parameter (nullptr if none).
- Parameters
-
[in] _owner reference on the parameter lister. [in] _name Static name of the parameter. [in] _defaultValue Default value of the parameter. [in] _description description of the parameter. [in] _setObs function of the class that opserve the change of the value
§ List() [2/2]
|
inline |
Create a parameter with List of element parameter (nullptr if none).
- Parameters
-
[in] _defaultValue Default value of the parameter.
§ ~List()
|
virtualdefault |
virtualisation of Destructor.
Member Function Documentation
§ add()
|
inline |
Add a value in the list of parameter.
- Parameters
-
[in] _value Value of the string [in] _name String of the value [in] _description Description of the parameter value
§ getInfo()
|
inlineoverridevirtual |
Description of the Propertys.
- Returns
- Descriptive information of the Property (for remote UI).
Implements eproperty::Property.
§ getListValue()
|
inlineoverridevirtual |
Specific for eproperty::List to get all the possible values.
- Returns
- Descriptive information of the Property (for remote UI).
Reimplemented from eproperty::Property.
§ getPropertyType()
|
inlineoverridevirtual |
Get the Property type of the class in string mode.
- Returns
- The string type of the Property.
Implements eproperty::Property.
§ remove()
|
inline |
Remove a value of the element availlable.
- Parameters
-
[in] _name Name of the value to remove
§ rename()
|
inline |
Rename a value of the property.
- Parameters
-
[in] _nameOld Old property name to replace [in] _nameNew New name of the property
§ set()
|
inlineoverridevirtual |
Set the value of the current parameter.
- Parameters
-
[in] _newVal New value of the parameter. (not set if out of range)
Reimplemented from eproperty::PropertyType< TYPE >.
§ setDirectCheck()
|
inlineoverridevirtual |
Set the value of the current parameter (check range and ... if needed).
- Note
- Only use by the owner of the property/
- Parameters
-
[in] _newVal New value to set
Reimplemented from eproperty::PropertyType< TYPE >.
§ setString()
|
inlineoverridevirtual |
Set a new value of the Property (with string interface).
- Parameters
-
[in] _newVal New value of the Propertys.
Implements eproperty::Property.
The documentation for this class was generated from the following file:
- framework/atria-soft/eproperty/eproperty/List.hpp