#include <Entry.hpp>
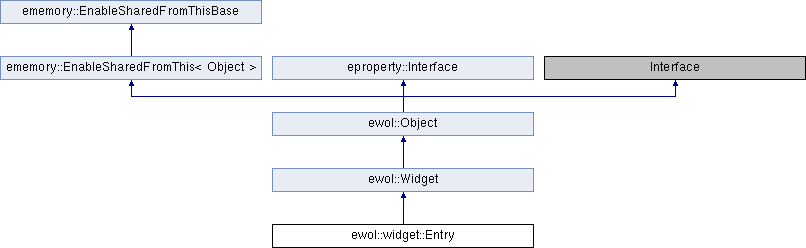
Public Member Functions | |
Entry () | |
void | init () override |
DECLARE_WIDGET_FACTORY (Entry, "Entry") | |
virtual | ~Entry () |
virtual void | copySelectionToClipBoard (enum gale::context::clipBoard::clipboardListe _clipboardID) |
virtual void | removeSelected () |
void | onRegenerateDisplay () override |
bool | onEventInput (const ewol::event::Input &_event) override |
bool | onEventEntry (const ewol::event::Entry &_event) override |
void | onEventClipboard (enum gale::context::clipBoard::clipboardListe _clipboardID) override |
void | calculateMinMaxSize () override |
![]() | |
virtual | ~Widget () |
virtual vec2 | relativePosition (const vec2 &_pos) |
virtual void | onChangeSize () |
virtual void | calculateSize () |
virtual vec2 | getSize () |
virtual void | setSize (const vec2 &_value) |
virtual vec2 | getCalculateMinSize () |
virtual vec2 | getCalculateMaxSize () |
virtual void | setOffset (const vec2 &_newVal) |
virtual const vec2 & | getOffset () |
virtual void | setZoom (float _newVal) |
virtual float | getZoom () |
virtual void | changeZoom (float _range) |
virtual void | setOrigin (const vec2 &_pos) |
virtual vec2 | getOrigin () |
void | setNoMinSize () |
virtual void | checkMinSize () |
void | setNoMaxSize () |
virtual void | checkMaxSize () |
virtual bvec2 | canExpand () |
const bvec2 & | canFill () |
virtual bool | getFocus () |
virtual bool | setFocus () |
virtual bool | rmFocus () |
virtual void | keepFocus () |
virtual int32_t | getMouseLimit () |
virtual void | setMouseLimit (int32_t _numberState) |
virtual bool | getKeyboardRepeate () |
virtual ewol::WidgetShared | getWidgetAtPos (const vec2 &_pos) |
virtual bool | systemEventInput (ewol::event::InputSystem &_event) |
virtual bool | systemEventEntry (ewol::event::EntrySystem &_event) |
virtual bool | onEventShortCut (const gale::key::Special &_special, char32_t _unicodeValue, enum gale::key::keyboard _kbMove, bool _isDown) |
virtual void | systemDraw (const DrawProperty &_displayProp) |
virtual void | grabCursor () |
virtual void | unGrabCursor () |
virtual bool | getGrabStatus () |
virtual void | setCursor (enum gale::context::cursor _newCursor) |
virtual enum gale::context::cursor | getCursor () |
virtual bool | loadXML (const exml::Element &_node) override |
void | requestUpdateSize () |
ewol::widget::Manager & | getWidgetManager () |
ememory::SharedPtr< ewol::widget::Windows > | getWindows () |
void | setAnnimationType (enum ewol::Widget::annimationMode _mode, const std::string &_type) |
void | setAnnimationTime (enum ewol::Widget::annimationMode _mode, float _time) |
bool | startAnnimation (enum ewol::Widget::annimationMode _mode) |
bool | stopAnnimation () |
![]() | |
DECLARE_FACTORY (Object) | |
virtual | ~Object () |
bool | objectHasBeenCorectlyInit () |
virtual void | destroy () |
bool | isDestroyed () const |
virtual void | setParent (const ewol::ObjectShared &_newParent) |
virtual void | removeParent () |
const char *const | getObjectType () const |
std::string | getTypeDescription () const |
bool | isTypeCompatible (const std::string &_type) const |
bool | getStatic () |
int32_t | getId () |
bool | propertySetOnWidgetNamed (const std::string &_objectName, const std::string &_config, const std::string &_value) |
virtual bool | storeXML (exml::Element &_node) const |
void | setStatusResource (bool _val) |
bool | getStatusResource () const |
virtual ewol::ObjectShared | getSubObjectNamed (const std::string &_objectName) |
![]() | |
ememory::SharedPtr< EMEMORY_TYPE > | sharedFromThis () |
const ememory::SharedPtr< EMEMORY_TYPE > | sharedFromThis () const |
ememory::WeakPtr< EMEMORY_TYPE > | weakFromThis () |
const ememory::WeakPtr< EMEMORY_TYPE > | weakFromThis () const |
Protected Member Functions | |
void | setInternalValue (const std::string &_newData) |
virtual void | markToUpdateTextPosition () |
virtual void | updateTextPosition () |
virtual void | updateCursorPosition (const vec2 &_pos, bool _Selection=false) |
void | onDraw () override |
void | onGetFocus () override |
void | onLostFocus () override |
virtual void | changeStatusIn (int32_t _newStatusId) |
void | periodicCall (const ewol::event::Time &_event) |
virtual void | onChangePropertyShaper () |
virtual void | onChangePropertyValue () |
virtual void | onChangePropertyMaxCharacter () |
virtual void | onChangePropertyRegex () |
virtual void | onChangePropertyTextWhenNothing () |
![]() | |
Widget () | |
virtual void | setKeyboardRepeate (bool _state) |
virtual void | showKeyboard () |
virtual void | hideKeyboard () |
virtual void | shortCutAdd (const std::string &_descriptiveString, const std::string &_message="") |
virtual void | shortCutClean () |
virtual void | shortCutRemove (const std::string &_message) |
virtual void | markToRedraw () |
virtual bool | needRedraw () |
void | addAnnimationType (enum ewol::Widget::annimationMode _mode, const char *_type) |
virtual bool | onStartAnnimation (enum ewol::Widget::annimationMode _mode) |
virtual void | onStopAnnimation () |
virtual void | onChangePropertyCanFocus () |
virtual void | onChangePropertyGravity () |
virtual void | onChangePropertyHide () |
virtual void | onChangePropertyFill () |
virtual void | onChangePropertyExpand () |
virtual void | onChangePropertyMaxSize () |
virtual void | onChangePropertyMinSize () |
![]() | |
Object () | |
virtual void | autoDestroy () |
virtual void | requestDestroyFromChild (const ewol::ObjectShared &_child) |
void | addObjectType (const char *_type) |
Protected Attributes | |
esignal::Connection | m_PCH |
![]() | |
vec2 | m_size |
vec2 | m_minSize |
vec2 | m_maxSize |
vec2 | m_offset |
float | m_zoom |
vec2 | m_origin |
bool | m_needRegenerateDisplay |
enum annimationMode | m_annimationMode |
float | m_annimationratio |
eproperty::List< int32_t > | propertyAnnimationTypeStart |
eproperty::Range< float > | propertyAnnimationTimeStart |
eproperty::List< int32_t > | propertyAnnimationTypeStop |
eproperty::Range< float > | propertyAnnimationTimeStop |
![]() | |
ewol::ObjectWeak | m_parent |
bool | m_destroy |
bool | m_static |
Additional Inherited Members | |
![]() | |
static ewol::object::Manager & | getObjectManager () |
static ewol::Context & | getContext () |
static ewol::ObjectShared | getObjectNamed (const std::string &_objectName) |
![]() | |
enum | annimationMode { annimationModeEnableAdd, annimationModeEnableRemove, annimationModeDisable } |
Detailed Description
Entry box display :
Constructor & Destructor Documentation
§ Entry()
ewol::widget::Entry::Entry | ( | ) |
Contuctor.
- Parameters
-
[in] _newData The USting that might be set in the Entry box (no event generation!!)
§ ~Entry()
|
virtual |
Destuctor.
Member Function Documentation
§ calculateMinMaxSize()
|
overridevirtual |
calculate the minimum and maximum size (need to estimate expend properties of the widget)
- Note
- : INTERNAL EWOL SYSTEM
Reimplemented from ewol::Widget.
§ copySelectionToClipBoard()
|
virtual |
Copy the selected data on the specify clipboard.
- Parameters
-
[in] _clipboardID Selected clipboard
§ markToUpdateTextPosition()
|
protectedvirtual |
informe the system thet the text change and the start position change
§ onDraw()
|
overrideprotectedvirtual |
Common widget drawing function (called by the drawing thread [Android, X11, ...])
Reimplemented from ewol::Widget.
§ onEventClipboard()
|
overridevirtual |
Event on a past event == > this event is asynchronous due to all system does not support direct getting datas.
- Note
- : need to have focus ...
- Parameters
-
[in] mode Mode of data requested
Reimplemented from ewol::Widget.
§ onEventEntry()
|
overridevirtual |
Entry event. represent the physical event :
- Keyboard (key event and move event)
- Accelerometer
- Joystick
- Parameters
-
[in] _event Event properties
- Returns
- true if the event has been used
- false if the event has not been used
Reimplemented from ewol::Widget.
§ onEventInput()
|
overridevirtual |
Event on an input of this Widget (finger, mouse, stilet)
- Parameters
-
[in] _event Event properties
- Returns
- true the event is used
- false the event is not used
Reimplemented from ewol::Widget.
§ onGetFocus()
|
overrideprotectedvirtual |
Event of the focus has been grep by the current widget.
Reimplemented from ewol::Widget.
§ onLostFocus()
|
overrideprotectedvirtual |
Event of the focus has been lost by the current widget.
Reimplemented from ewol::Widget.
§ onRegenerateDisplay()
|
overridevirtual |
Event generated when a redraw is needed.
Reimplemented from ewol::Widget.
§ periodicCall()
|
protected |
Periodic call to update grapgic display.
- Parameters
-
[in] _event Time generic event
§ removeSelected()
|
virtual |
remove the selected area
- Note
- This request a regeneration of the display
§ setInternalValue()
|
protected |
internal check the value with RegExp checking
- Parameters
-
[in] _newData The new string to display
§ updateCursorPosition()
|
protectedvirtual |
change the cursor position with the curent position requested on the display
- Parameters
-
[in] _pos Absolute position of the event
- Note
- The display is automaticly requested when change apear.
§ updateTextPosition()
|
protectedvirtual |
update the display position start == > depending of the position of the Cursor and the size of the Data inside m_displayStartPosition < == updated
Member Data Documentation
§ m_PCH
|
protected |
Periodic call handle to remove it when needed.
§ propertyMaxCharacter
eproperty::Range<int32_t> ewol::widget::Entry::propertyMaxCharacter |
number max of xharacter in the list
§ propertyRegex
eproperty::Value<std::string> ewol::widget::Entry::propertyRegex |
regular expression value
§ propertyTextWhenNothing
eproperty::Value<std::string> ewol::widget::Entry::propertyTextWhenNothing |
Text to display when nothing in in the entry (decorated text...)
§ propertyValue
eproperty::Value<std::string> ewol::widget::Entry::propertyValue |
string that must be displayed
§ signalClick
esignal::Signal ewol::widget::Entry::signalClick |
bang on click the entry box
§ signalEnter
esignal::Signal<std::string> ewol::widget::Entry::signalEnter |
Enter key is pressed.
§ signalModify
esignal::Signal<std::string> ewol::widget::Entry::signalModify |
data change
The documentation for this class was generated from the following file:
- framework/atria-soft/ewol/ewol/widget/Entry.hpp