#include <Widget.hpp>
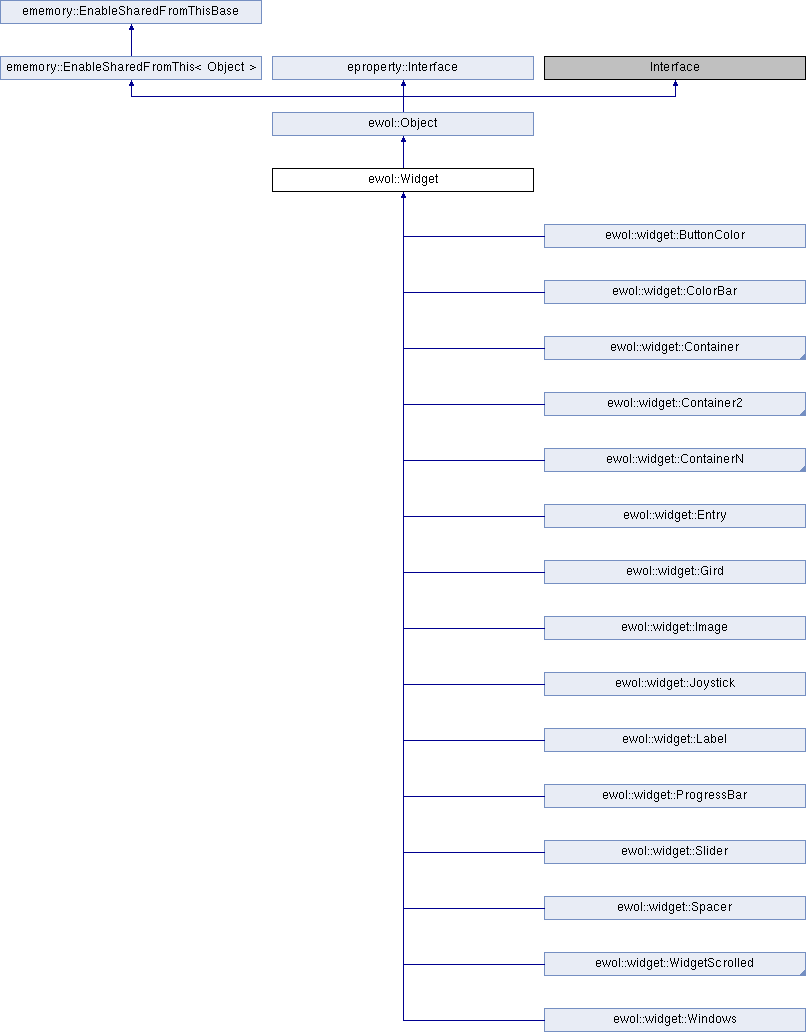
Public Member Functions | |
virtual | ~Widget () |
virtual vec2 | relativePosition (const vec2 &_pos) |
virtual void | onChangeSize () |
virtual void | calculateSize () |
virtual vec2 | getSize () |
virtual void | setSize (const vec2 &_value) |
virtual void | calculateMinMaxSize () |
virtual vec2 | getCalculateMinSize () |
virtual vec2 | getCalculateMaxSize () |
virtual void | setOffset (const vec2 &_newVal) |
virtual const vec2 & | getOffset () |
virtual void | setZoom (float _newVal) |
virtual float | getZoom () |
virtual void | changeZoom (float _range) |
virtual void | setOrigin (const vec2 &_pos) |
virtual vec2 | getOrigin () |
void | setNoMinSize () |
virtual void | checkMinSize () |
void | setNoMaxSize () |
virtual void | checkMaxSize () |
virtual bvec2 | canExpand () |
const bvec2 & | canFill () |
virtual bool | getFocus () |
virtual bool | setFocus () |
virtual bool | rmFocus () |
virtual void | keepFocus () |
virtual int32_t | getMouseLimit () |
virtual void | setMouseLimit (int32_t _numberState) |
virtual bool | getKeyboardRepeate () |
virtual ewol::WidgetShared | getWidgetAtPos (const vec2 &_pos) |
virtual bool | systemEventInput (ewol::event::InputSystem &_event) |
virtual bool | systemEventEntry (ewol::event::EntrySystem &_event) |
virtual void | onEventClipboard (enum gale::context::clipBoard::clipboardListe _clipboardID) |
virtual bool | onEventShortCut (const gale::key::Special &_special, char32_t _unicodeValue, enum gale::key::keyboard _kbMove, bool _isDown) |
virtual void | systemDraw (const DrawProperty &_displayProp) |
virtual void | onRegenerateDisplay () |
virtual void | grabCursor () |
virtual void | unGrabCursor () |
virtual bool | getGrabStatus () |
virtual void | setCursor (enum gale::context::cursor _newCursor) |
virtual enum gale::context::cursor | getCursor () |
virtual bool | loadXML (const exml::Element &_node) override |
void | requestUpdateSize () |
ewol::widget::Manager & | getWidgetManager () |
ememory::SharedPtr< ewol::widget::Windows > | getWindows () |
void | setAnnimationType (enum ewol::Widget::annimationMode _mode, const std::string &_type) |
void | setAnnimationTime (enum ewol::Widget::annimationMode _mode, float _time) |
bool | startAnnimation (enum ewol::Widget::annimationMode _mode) |
bool | stopAnnimation () |
![]() | |
DECLARE_FACTORY (Object) | |
virtual | ~Object () |
bool | objectHasBeenCorectlyInit () |
virtual void | destroy () |
bool | isDestroyed () const |
virtual void | setParent (const ewol::ObjectShared &_newParent) |
virtual void | removeParent () |
const char *const | getObjectType () const |
std::string | getTypeDescription () const |
bool | isTypeCompatible (const std::string &_type) const |
bool | getStatic () |
int32_t | getId () |
bool | propertySetOnWidgetNamed (const std::string &_objectName, const std::string &_config, const std::string &_value) |
virtual bool | storeXML (exml::Element &_node) const |
void | setStatusResource (bool _val) |
bool | getStatusResource () const |
virtual ewol::ObjectShared | getSubObjectNamed (const std::string &_objectName) |
![]() | |
ememory::SharedPtr< EMEMORY_TYPE > | sharedFromThis () |
const ememory::SharedPtr< EMEMORY_TYPE > | sharedFromThis () const |
ememory::WeakPtr< EMEMORY_TYPE > | weakFromThis () |
const ememory::WeakPtr< EMEMORY_TYPE > | weakFromThis () const |
Public Attributes | |
eproperty::Value< gale::Dimension > | propertyMinSize |
eproperty::Value< gale::Dimension > | propertyMaxSize |
eproperty::Value< bvec2 > | propertyExpand |
eproperty::Value< bvec2 > | propertyFill |
eproperty::Value< bool > | propertyHide |
eproperty::List< enum ewol::gravity > | propertyGravity |
eproperty::Value< bool > | propertyCanFocus |
esignal::Signal< std::string > | signalShortcut |
esignal::Signal | signalAnnimationStart |
esignal::Signal< float > | signalAnnimationRatio |
esignal::Signal | signalAnnimationStop |
![]() | |
eproperty::Value< std::string > | propertyName |
![]() | |
eproperty::InterfaceData | properties |
Protected Types | |
enum | annimationMode { annimationModeEnableAdd, annimationModeEnableRemove, annimationModeDisable } |
Protected Member Functions | |
Widget () | |
virtual void | onGetFocus () |
virtual void | onLostFocus () |
virtual void | setKeyboardRepeate (bool _state) |
virtual void | showKeyboard () |
virtual void | hideKeyboard () |
virtual bool | onEventInput (const ewol::event::Input &_event) |
virtual bool | onEventEntry (const ewol::event::Entry &_event) |
virtual void | shortCutAdd (const std::string &_descriptiveString, const std::string &_message="") |
virtual void | shortCutClean () |
virtual void | shortCutRemove (const std::string &_message) |
virtual void | markToRedraw () |
virtual bool | needRedraw () |
virtual void | onDraw () |
void | addAnnimationType (enum ewol::Widget::annimationMode _mode, const char *_type) |
virtual bool | onStartAnnimation (enum ewol::Widget::annimationMode _mode) |
virtual void | onStopAnnimation () |
virtual void | onChangePropertyCanFocus () |
virtual void | onChangePropertyGravity () |
virtual void | onChangePropertyHide () |
virtual void | onChangePropertyFill () |
virtual void | onChangePropertyExpand () |
virtual void | onChangePropertyMaxSize () |
virtual void | onChangePropertyMinSize () |
![]() | |
Object () | |
virtual void | init () |
virtual void | autoDestroy () |
virtual void | requestDestroyFromChild (const ewol::ObjectShared &_child) |
void | addObjectType (const char *_type) |
Protected Attributes | |
vec2 | m_size |
vec2 | m_minSize |
vec2 | m_maxSize |
vec2 | m_offset |
float | m_zoom |
vec2 | m_origin |
bool | m_needRegenerateDisplay |
enum annimationMode | m_annimationMode |
float | m_annimationratio |
eproperty::List< int32_t > | propertyAnnimationTypeStart |
eproperty::Range< float > | propertyAnnimationTimeStart |
eproperty::List< int32_t > | propertyAnnimationTypeStop |
eproperty::Range< float > | propertyAnnimationTimeStop |
![]() | |
ewol::ObjectWeak | m_parent |
bool | m_destroy |
bool | m_static |
Additional Inherited Members | |
![]() | |
static ewol::object::Manager & | getObjectManager () |
static ewol::Context & | getContext () |
static ewol::ObjectShared | getObjectNamed (const std::string &_objectName) |
Detailed Description
Widget class is the main widget interface, it hase some generic properties: :** known his parent :** Can be display at a special position with a special scale :** Can get focus :** Receive Event (keyboard / mouse / ...)
Constructor & Destructor Documentation
§ Widget()
|
protected |
Constructor of the widget classes.
- Returns
- (no execption generated (not managed in embended platform))
§ ~Widget()
|
virtual |
Destructor of the widget classes.
Member Function Documentation
§ addAnnimationType()
|
protected |
Add a annimation type capabilities of this widget.
- Parameters
-
[in] _mode Configuring mode. [in] _type Type of the annimation.
§ calculateMinMaxSize()
|
virtual |
calculate the minimum and maximum size (need to estimate expend properties of the widget)
- Note
- : INTERNAL EWOL SYSTEM
Reimplemented in ewol::widget::Container2, ewol::widget::Gird, ewol::widget::Entry, ewol::widget::ContainerN, ewol::widget::Button, ewol::widget::ParameterList, ewol::widget::CheckBox, ewol::widget::Container, ewol::widget::Image, ewol::widget::ContextMenu, ewol::widget::Sizer, ewol::widget::ButtonColor, ewol::widget::Label, ewol::widget::Scroll, ewol::widget::Slider, ewol::widget::ProgressBar, ewol::widget::ColorBar, and ewol::widget::List.
§ canExpand()
|
virtual |
get the expend capabilities (x&y)
- Returns
- 2D boolean repensent the capacity to expend
- Note
- : INTERNAL EWOL SYSTEM
Reimplemented in ewol::widget::ContainerN.
§ canFill()
const bvec2& ewol::Widget::canFill | ( | ) |
get the filling capabilities x&y
- Returns
- bvec2 repensent the capacity to x&y filling
- Note
- : INTERNAL EWOL SYSTEM
§ changeZoom()
|
inlinevirtual |
Change Zoom property.
- Parameters
-
[in] _range Range of the zoom change.
§ checkMaxSize()
|
virtual |
Check if the current max size is compatible with the user maximum size If it is not the user maximum size will overWrite the maximum size set.
- Note
- : INTERNAL EWOL SYSTEM
§ checkMinSize()
|
virtual |
Check if the current min size is compatible with the user minimum size If it is not the user minimum size will overWrite the minimum size set.
- Note
- : INTERNAL EWOL SYSTEM
§ getCalculateMaxSize()
|
virtual |
get the widget maximum size calculated
- Returns
- Requested size
- Note
- : INTERNAL EWOL SYSTEM
§ getCalculateMinSize()
|
virtual |
get the widget minimum size calculated
- Returns
- Requested size
- Note
- : INTERNAL EWOL SYSTEM
§ getCursor()
|
virtual |
get the currrent cursor.
- Returns
- the type of the cursor.
§ getFocus()
|
inlinevirtual |
get the focus state of the widget
- Returns
- focus state
§ getGrabStatus()
|
virtual |
get the grabbing status of the cursor.
- Returns
- true if the cursor is curently grabbed
§ getKeyboardRepeate()
|
inlinevirtual |
get the keyboard repeating event supporting.
- Returns
- true : the event can be repeated.
- false : the event must not be repeated.
§ getMouseLimit()
|
inlinevirtual |
get the number of mouse event supported
- Returns
- return the number of event that the mouse supported [0..3]
§ getOffset()
|
inlinevirtual |
get the offset property of the widget.
- Returns
- The current offset value.
§ getOrigin()
|
virtual |
get the origin (obsolute position in the windows)
- Returns
- coordonate of the origin requested
§ getSize()
|
virtual |
get the widget size
- Returns
- Requested size
- Note
- : INTERNAL EWOL SYSTEM
§ getWidgetAtPos()
|
inlinevirtual |
get the widget at the specific windows absolute position
- Parameters
-
[in] _pos gAbsolute position of the requested widget knowledge
- Returns
- nullptr No widget found
- pointer on the widget found
- Note
- : INTERNAL EWOL SYSTEM
Reimplemented in ewol::widget::Gird, ewol::widget::ContainerN, ewol::widget::WSlider, ewol::widget::Windows, ewol::widget::Container, ewol::widget::ContextMenu, ewol::widget::PopUp, ewol::widget::Scroll, ewol::widget::Spacer, and ewol::widget::Layer.
§ getWidgetManager()
ewol::widget::Manager& ewol::Widget::getWidgetManager | ( | ) |
get the current Widget Manager
§ getWindows()
ememory::SharedPtr<ewol::widget::Windows> ewol::Widget::getWindows | ( | ) |
get the curent Windows
§ getZoom()
|
virtual |
get the zoom property of the widget
- Returns
- the current zoom value
§ grabCursor()
|
virtual |
Grab the cursor : This get all the mouvement of the mouse in PC mode, and generate an ofset instead of a position.
- Note
- : the generation of the offset is due to the fact the cursor position is forced at the center of the widget.
- This done nothing in "Finger" or "Stylet" mode.
§ hideKeyboard()
|
protectedvirtual |
Hide the virtual keyboard (if needed)
§ keepFocus()
|
virtual |
keep the focus on this widget == > this remove the previous focus on all other widget
§ loadXML()
|
overridevirtual |
load properties with an XML node.
- Parameters
-
[in] _node Pointer on the tinyXML node.
- Returns
- true : All has been done corectly.
- false : An error occured.
Reimplemented from ewol::Object.
Reimplemented in ewol::widget::Container2, ewol::widget::ContainerN, ewol::widget::SpinBase, ewol::widget::Container, ewol::widget::Image, ewol::widget::Label, and ewol::widget::Select.
§ markToRedraw()
|
protectedvirtual |
The widget mark itself that it need to regenerate the nest time.
§ needRedraw()
|
inlineprotectedvirtual |
get the need of the redrawing of the widget and reset it to false
- Returns
- true if we need to redraw
- false if we have no need to redraw
§ onChangeSize()
|
virtual |
Parent have set the size and the origin. the container need to update the subwidget property.
- Note
- : INTERNAL EWOL SYSTEM
Reimplemented in ewol::widget::Container2, ewol::widget::Gird, ewol::widget::ContainerN, ewol::widget::Button, ewol::widget::Windows, ewol::widget::WSlider, ewol::widget::CheckBox, ewol::widget::Container, ewol::widget::ContextMenu, ewol::widget::PopUp, ewol::widget::Sizer, and ewol::widget::Scroll.
§ onDraw()
|
inlineprotectedvirtual |
Common widget drawing function (called by the drawing thread [Android, X11, ...])
Reimplemented in ewol::widget::Entry, ewol::widget::WidgetScrolled, ewol::widget::Button, ewol::widget::List, ewol::widget::ParameterList, ewol::widget::CheckBox, ewol::widget::Image, ewol::widget::Scroll, ewol::widget::ContextMenu, ewol::widget::ButtonColor, ewol::widget::Label, ewol::widget::Slider, ewol::widget::PopUp, ewol::widget::Spacer, ewol::widget::ProgressBar, and ewol::widget::ColorBar.
§ onEventClipboard()
|
inlinevirtual |
Event on a past event == > this event is asynchronous due to all system does not support direct getting datas.
- Note
- : need to have focus ...
- Parameters
-
[in] mode Mode of data requested
Reimplemented in ewol::widget::Entry.
§ onEventEntry()
|
inlineprotectedvirtual |
Entry event. represent the physical event :
- Keyboard (key event and move event)
- Accelerometer
- Joystick
- Parameters
-
[in] _event Event properties
- Returns
- true if the event has been used
- false if the event has not been used
Reimplemented in ewol::widget::Entry, ewol::widget::Button, and ewol::widget::CheckBox.
§ onEventInput()
|
inlineprotectedvirtual |
Event on an input of this Widget (finger, mouse, stilet)
- Parameters
-
[in] _event Event properties
- Returns
- true the event is used
- false the event is not used
Reimplemented in ewol::widget::Entry, ewol::widget::Button, ewol::widget::Joystick, ewol::widget::WidgetScrolled, ewol::widget::List, ewol::widget::ParameterList, ewol::widget::CheckBox, ewol::widget::Image, ewol::widget::ContextMenu, ewol::widget::ButtonColor, ewol::widget::PopUp, ewol::widget::Label, ewol::widget::Scroll, ewol::widget::Slider, and ewol::widget::ColorBar.
§ onEventShortCut()
|
virtual |
Event on a short-cut of this Widget (in case of return false, the event on the keyevent will arrive in the function onEventKb).
- Parameters
-
[in] _special All the special kay pressed at this time. [in] _unicodeValue Key pressed by the user not used if the kbMove!=ewol::EVENT_KB_MOVE_TYPE_NONE. [in] _kbMove Special key of the keyboard.
- Returns
- true if the event has been used.
- false if the event has not been used.
- Note
- To prevent some error when you get an event get it if it is down and Up ... == > like this it could not generate some ununderstanding error.
§ onGetFocus()
|
inlineprotectedvirtual |
Event of the focus has been grep by the current widget.
Reimplemented in ewol::widget::Entry, ewol::widget::FileChooser, ewol::widget::List, and ewol::widget::ParameterList.
§ onLostFocus()
|
inlineprotectedvirtual |
Event of the focus has been lost by the current widget.
Reimplemented in ewol::widget::Entry, ewol::widget::Button, ewol::widget::List, and ewol::widget::ParameterList.
§ onRegenerateDisplay()
|
inlinevirtual |
Event generated when a redraw is needed.
Reimplemented in ewol::widget::Container2, ewol::widget::Gird, ewol::widget::Entry, ewol::widget::ContainerN, ewol::widget::Button, ewol::widget::Joystick, ewol::widget::WidgetScrolled, ewol::widget::List, ewol::widget::WSlider, ewol::widget::ParameterList, ewol::widget::CheckBox, ewol::widget::Windows, ewol::widget::Image, ewol::widget::Container, ewol::widget::ContextMenu, ewol::widget::ButtonColor, ewol::widget::PopUp, ewol::widget::Label, ewol::widget::Scroll, ewol::widget::Slider, ewol::widget::Spacer, ewol::widget::ProgressBar, and ewol::widget::ColorBar.
§ onStartAnnimation()
|
inlineprotectedvirtual |
Event when start the annimation.
- Parameters
-
[in] _mode Configuring mode.
- Returns
- true need to add periodic call.
Reimplemented in ewol::widget::PopUp.
§ onStopAnnimation()
|
inlineprotectedvirtual |
Event when Stop the annimation.
Reimplemented in ewol::widget::PopUp.
§ relativePosition()
convert the absolute position in the local Position (Relative)
- Parameters
-
[in] _pos Absolute position that you request convertion
- Returns
- the relative position
§ requestUpdateSize()
void ewol::Widget::requestUpdateSize | ( | ) |
need to be call When the size of the current widget have change ==> this force the system to recalculate all the widget positions
§ rmFocus()
|
virtual |
remove the focus on this widget
- Returns
- return true if the widget have release his focus (if he has it)
§ setAnnimationTime()
void ewol::Widget::setAnnimationTime | ( | enum ewol::Widget::annimationMode | _mode, |
float | _time | ||
) |
set a annimation time to produce.
- Parameters
-
[in] _mode Configuring mode. [in] _time Time in second of the annimation display
§ setAnnimationType()
void ewol::Widget::setAnnimationType | ( | enum ewol::Widget::annimationMode | _mode, |
const std::string & | _type | ||
) |
set a annimation type.
- Parameters
-
[in] _mode Configuring mode. [in] _type type of the annimation
§ setCursor()
|
virtual |
set the cursor display type.
- Parameters
-
[in] _newCursor selected new cursor.
§ setFocus()
|
virtual |
set focus on this widget
- Returns
- return true if the widget keep the focus
§ setKeyboardRepeate()
|
inlineprotectedvirtual |
set the keyboard repeating event supporting.
- Parameters
-
[in] _state The repeating status (true: enable, false disable).
§ setMouseLimit()
|
inlinevirtual |
get the number of mouse event supported
- Parameters
-
[in] _numberState The number of event that the mouse supported [0..3]
§ setNoMaxSize()
void ewol::Widget::setNoMaxSize | ( | ) |
User set No maximum size.
§ setNoMinSize()
void ewol::Widget::setNoMinSize | ( | ) |
User set No minimum size.
§ setOffset()
|
virtual |
set the zoom property of the widget.
- Parameters
-
[in] _newVal offset value.
Reimplemented in ewol::widget::Container2, ewol::widget::ContainerN, and ewol::widget::Container.
§ setOrigin()
|
virtual |
set origin at the widget (must be an parrent widget that set this parameter). This represent the absolute origin in the program windows
- Parameters
-
[in] _pos Position of the origin
- Note
- : INTERNAL EWOL SYSTEM
§ setSize()
|
inlinevirtual |
set the widget size
- Returns
- Requested size
- Note
- : INTERNAL EWOL SYSTEM Do not modify the size yourself: calculation is complex and need knowledge of around widget
§ setZoom()
|
virtual |
set the zoom property of the widget
- Parameters
-
[in] _newVal newZoom value
§ shortCutAdd()
|
protectedvirtual |
add a specific shortcut with his description
- Parameters
-
[in] _descriptiveString Description string of the shortcut [in] _message massage to generate (or shortcut name)
§ shortCutClean()
|
protectedvirtual |
remove all curent shortCut
§ shortCutRemove()
|
protectedvirtual |
remove a specific shortCut whith his event name
- Parameters
-
[in] _message renerated event name
§ showKeyboard()
|
protectedvirtual |
display the virtual keyboard (if needed)
§ startAnnimation()
bool ewol::Widget::startAnnimation | ( | enum ewol::Widget::annimationMode | _mode | ) |
Start the annimation.
- Parameters
-
[in] _mode Configuring mode.
- Returns
- true if an annimation will be started, false ==> no annimation and no event
§ stopAnnimation()
bool ewol::Widget::stopAnnimation | ( | ) |
Stop/Break the annimation.
- Returns
- true if an annimation will be stoped, false ==> no curent annimation and no event wil be generated
§ systemDraw()
|
virtual |
{SYSTEM} extern interface to request a draw ... (called by the drawing thread [Android, X11, ...]) This function generate a clipping with the viewport openGL system. Like this a widget draw can not draw over an other widget
- Note
- This function is virtual for the scrolled widget, and the more complicated openGl widget
- Parameters
-
[in] _displayProp properties of the current display
- Note
- : INTERNAL EWOL SYSTEM
Reimplemented in ewol::widget::Container2, ewol::widget::Gird, ewol::widget::ContainerN, ewol::widget::WidgetScrolled, ewol::widget::WSlider, ewol::widget::Windows, ewol::widget::Container, ewol::widget::Scroll, and ewol::widget::PopUp.
§ systemEventEntry()
|
virtual |
{SYSTEM} Entry event (only meta widget might overwrite this function).
- Parameters
-
[in] _event Event properties
- Returns
- true if the event has been used
- false if the event has not been used
§ systemEventInput()
|
virtual |
{SYSTEM} system event input (only meta widget might overwrite this function).
- Parameters
-
[in] _event Event properties
- Returns
- true the event is used
- false the event is not used
§ unGrabCursor()
|
virtual |
Un-Grab the cursor (default mode cursor offset)
Member Data Documentation
§ m_annimationMode
|
protected |
true when the annimation is started
§ m_annimationratio
|
protected |
Ratio of the annimation [0..1].
§ m_maxSize
|
protected |
internal: maximum size of the widget
§ m_minSize
|
protected |
internal: minimum size of the widget
§ m_needRegenerateDisplay
|
protected |
the display might be done the next regeneration
§ m_offset
|
protected |
Offset of the display in the viewport.
§ m_origin
|
protected |
internal ... I do not really known how if can use it ...
§ m_size
|
protected |
internal: current size of the widget
§ m_zoom
|
protected |
generic widget zoom
§ propertyAnnimationTimeStart
|
protected |
time to produce start annimation
§ propertyAnnimationTimeStop
|
protected |
time to produce start annimation
§ propertyAnnimationTypeStart
|
protected |
type of start annimation
§ propertyAnnimationTypeStop
|
protected |
type of start annimation
§ propertyCanFocus
eproperty::Value<bool> ewol::Widget::propertyCanFocus |
the focus can be done on this widget
§ propertyExpand
eproperty::Value<bvec2> ewol::Widget::propertyExpand |
the widget will expand if possible
§ propertyFill
eproperty::Value<bvec2> ewol::Widget::propertyFill |
the widget will fill all the space provided by the parrent.
§ propertyGravity
eproperty::List<enum ewol::gravity> ewol::Widget::propertyGravity |
Gravity of the widget.
§ propertyHide
eproperty::Value<bool> ewol::Widget::propertyHide |
hide a widget on the display
§ propertyMaxSize
eproperty::Value<gale::Dimension> ewol::Widget::propertyMaxSize |
user define the maximum size of the widget
§ propertyMinSize
eproperty::Value<gale::Dimension> ewol::Widget::propertyMinSize |
user define the minimum size of the widget
§ signalAnnimationRatio
esignal::Signal<float> ewol::Widget::signalAnnimationRatio |
event when % of annimation change (integer)
§ signalAnnimationStart
esignal::Signal ewol::Widget::signalAnnimationStart |
event when start annimation
§ signalAnnimationStop
esignal::Signal ewol::Widget::signalAnnimationStop |
event when stop annimation
§ signalShortcut
esignal::Signal<std::string> ewol::Widget::signalShortcut |
signal handle of the message
The documentation for this class was generated from the following file:
- framework/atria-soft/ewol/ewol/widget/Widget.hpp