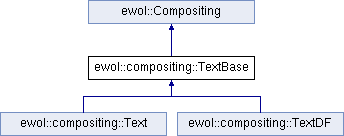
Public Member Functions | |
virtual ewol::compositing::Drawing & | getDrawing () |
virtual void | loadProgram (const std::string &_shaderName) |
TextBase (const std::string &_shaderName="{ewol}DATA:text.prog", bool _loadProgram=true) | |
virtual | ~TextBase () |
void | translate (const vec3 &_vect) |
void | rotate (const vec3 &_vect, float _angle) |
void | scale (const vec3 &_vect) |
void | draw (bool _disableDepthTest=true) |
void | draw (const mat4 &_transformationMatrix, bool _enableDepthTest=false) |
virtual void | drawD (bool _disableDepthTest)=0 |
virtual void | drawMT (const mat4 &_transformationMatrix, bool _enableDepthTest)=0 |
virtual void | clear () |
virtual void | reset () |
const vec3 & | getPos () |
void | setPos (const vec3 &_pos) |
void | setPos (const vec2 &_pos) |
void | setRelPos (const vec3 &_pos) |
void | setRelPos (const vec2 &_pos) |
void | setDefaultColorBg (const etk::Color<> &_color) |
void | setDefaultColorFg (const etk::Color<> &_color) |
void | setColor (const etk::Color<> &_color) |
void | setColorBg (const etk::Color<> &_color) |
void | setClippingWidth (const vec3 &_pos, const vec3 &_width) |
void | setClippingWidth (const vec2 &_pos, const vec2 &_width) |
void | setClipping (const vec3 &_pos, const vec3 &_posEnd) |
void | setClipping (const vec2 &_pos, const vec2 &_posEnd) |
void | setClippingMode (bool _newMode) |
virtual void | setFontSize (int32_t _fontSize)=0 |
virtual void | setFontName (const std::string &_fontName)=0 |
virtual void | setFont (std::string _fontName, int32_t _fontSize)=0 |
virtual void | setFontMode (enum ewol::font::mode _mode)=0 |
enum ewol::font::mode | getFontMode () |
virtual float | getHeight ()=0 |
virtual float | getSize ()=0 |
virtual ewol::GlyphProperty * | getGlyphPointer (char32_t _charcode)=0 |
void | setFontBold (bool _status) |
void | setFontItalic (bool _status) |
void | setKerningMode (bool _newMode) |
void | print (const std::string &_text) |
void | print (const std::u32string &_text) |
void | printDecorated (const std::string &_text) |
void | printDecorated (const std::u32string &_text) |
void | printHTML (const std::string &_text) |
void | printHTML (const std::u32string &_text) |
void | print (const std::string &_text, const std::vector< TextDecoration > &_decoration) |
void | print (const std::u32string &_text, const std::vector< TextDecoration > &_decoration) |
virtual void | printChar (const char32_t &_charcode)=0 |
void | forceLineReturn () |
void | setTextAlignement (float _startTextpos, float _stopTextPos, enum ewol::compositing::aligneMode _alignement=ewol::compositing::alignDisable) |
void | disableAlignement () |
enum ewol::compositing::aligneMode | getAlignement () |
vec3 | calculateSizeHTML (const std::string &_text) |
vec3 | calculateSizeHTML (const std::u32string &_text) |
vec3 | calculateSizeDecorated (const std::string &_text) |
vec3 | calculateSizeDecorated (const std::u32string &_text) |
vec3 | calculateSize (const std::string &_text) |
vec3 | calculateSize (const std::u32string &_text) |
vec3 | calculateSize (const char32_t &_charcode) |
void | printCursor (bool _isInsertMode, float _cursorSize=20.0f) |
void | disableCursor () |
void | setCursorPos (int32_t _cursorPos) |
void | setCursorSelection (int32_t _cursorPos, int32_t _selectionStartPos) |
void | setSelectionColor (const etk::Color<> &_color) |
void | setCursorColor (const etk::Color<> &_color) |
![]() | |
Compositing () | |
virtual | ~Compositing () |
virtual void | resetMatrix () |
virtual void | setMatrix (const mat4 &_mat) |
Protected Member Functions | |
void | parseHtmlNode (const exml::Element &_element) |
virtual vec3 | calculateSizeChar (const char32_t &_charcode)=0 |
bool | extrapolateLastId (const std::string &_text, const int32_t _start, int32_t &_stop, int32_t &_space, int32_t &_freeSpace) |
bool | extrapolateLastId (const std::u32string &_text, const int32_t _start, int32_t &_stop, int32_t &_space, int32_t &_freeSpace) |
void | htmlAddData (const std::u32string &_data) |
void | htmlFlush () |
Constructor & Destructor Documentation
§ TextBase()
ewol::compositing::TextBase::TextBase | ( | const std::string & | _shaderName = "{ewol}DATA:text.prog" , |
bool | _loadProgram = true |
||
) |
generic constructor
§ ~TextBase()
|
virtual |
generic destructor
Member Function Documentation
§ calculateSize() [1/3]
vec3 ewol::compositing::TextBase::calculateSize | ( | const std::string & | _text | ) |
calculate a theoric text size
- Parameters
-
[in] _text The string to calculate dimention.
- Returns
- The theoric size used.
§ calculateSize() [2/3]
vec3 ewol::compositing::TextBase::calculateSize | ( | const std::u32string & | _text | ) |
§ calculateSize() [3/3]
|
inline |
calculate a theoric charcode size
- Parameters
-
[in] _charcode The µUnicode value to calculate dimention.
- Returns
- The theoric size used.
§ calculateSizeChar()
|
protectedpure virtual |
Implemented in ewol::compositing::TextDF, and ewol::compositing::Text.
§ calculateSizeDecorated() [1/2]
vec3 ewol::compositing::TextBase::calculateSizeDecorated | ( | const std::string & | _text | ) |
calculate a theoric text size
- Parameters
-
[in] _text The string to calculate dimention.
- Returns
- The theoric size used.
§ calculateSizeDecorated() [2/2]
vec3 ewol::compositing::TextBase::calculateSizeDecorated | ( | const std::u32string & | _text | ) |
§ calculateSizeHTML() [1/2]
vec3 ewol::compositing::TextBase::calculateSizeHTML | ( | const std::string & | _text | ) |
calculate a theoric text size
- Parameters
-
[in] _text The string to calculate dimention.
- Returns
- The theoric size used.
§ calculateSizeHTML() [2/2]
vec3 ewol::compositing::TextBase::calculateSizeHTML | ( | const std::u32string & | _text | ) |
§ clear()
|
virtual |
clear all the registered element in the current element
Reimplemented from ewol::Compositing.
Reimplemented in ewol::compositing::TextDF.
§ disableAlignement()
void ewol::compositing::TextBase::disableAlignement | ( | ) |
disable the alignement system
§ disableCursor()
void ewol::compositing::TextBase::disableCursor | ( | ) |
remove the cursor display
§ draw() [1/2]
|
inlinevirtual |
draw All the refistered text in the current element on openGL
Implements ewol::Compositing.
§ draw() [2/2]
|
inline |
§ drawD()
|
pure virtual |
draw All the refistered text in the current element on openGL
Implemented in ewol::compositing::TextDF, and ewol::compositing::Text.
§ drawMT()
|
pure virtual |
Implemented in ewol::compositing::TextDF, and ewol::compositing::Text.
§ extrapolateLastId() [1/2]
|
protected |
calculate the element number that is the first out the alignement range (start at the specify ID, and use start pos with current one)
- Parameters
-
[in] _text The string that might be parsed. [in] _start The first elemnt that might be used to calculate. [out] _stop The last Id availlable in the current string. [out] _space Number of space in the string. [out] _freespace This represent the number of pixel present in the right white space.
- Returns
- true if the rifht has free space that can be use for jystify.
-
false if we find '
'
§ extrapolateLastId() [2/2]
|
protected |
§ forceLineReturn()
void ewol::compositing::TextBase::forceLineReturn | ( | ) |
This generate the line return == > it return to the alignement position start and at the correct line position ==> it might be use to not know the line height.
§ getAlignement()
enum ewol::compositing::aligneMode ewol::compositing::TextBase::getAlignement | ( | ) |
get the current alignement property
- Returns
- the curent alignement type
§ getFontMode()
|
inline |
get the current font mode
- Returns
- The font mode applied
§ getPos()
|
inline |
get the current display position (sometime needed in the gui control)
- Returns
- the current position.
§ htmlAddData()
|
protected |
add a line with the current m_htmlDecoTmp decoration
- Parameters
-
[in] _data The cuurent data to add.
§ htmlFlush()
|
protected |
draw the current line
§ loadProgram()
|
virtual |
load the openGL program and get all the ID needed
Reimplemented in ewol::compositing::TextDF.
§ parseHtmlNode()
|
protected |
This parse a tinyXML node (void pointer to permit to hide tiny XML in include).
- Parameters
-
[in] _element the exml element.
§ print() [1/4]
void ewol::compositing::TextBase::print | ( | const std::string & | _text | ) |
display a compleat string in the current element.
- Parameters
-
[in] _text The string to display.
§ print() [2/4]
void ewol::compositing::TextBase::print | ( | const std::u32string & | _text | ) |
§ print() [3/4]
void ewol::compositing::TextBase::print | ( | const std::string & | _text, |
const std::vector< TextDecoration > & | _decoration | ||
) |
display a compleat string in the current element whith specific decorations (advence mode).
- Parameters
-
[in] _text The string to display. [in] _decoration The text decoration for the text that might be display (if the vector is smaller, the last parameter is get)
§ print() [4/4]
void ewol::compositing::TextBase::print | ( | const std::u32string & | _text, |
const std::vector< TextDecoration > & | _decoration | ||
) |
§ printChar()
|
pure virtual |
display the current char in the current element (note that the kerning is availlable if the position is not changed)
- Parameters
-
[in] _charcode Char that might be dispalyed
Implemented in ewol::compositing::TextDF, and ewol::compositing::Text.
§ printCursor()
void ewol::compositing::TextBase::printCursor | ( | bool | _isInsertMode, |
float | _cursorSize = 20.0f |
||
) |
draw a cursor at the specify position
- Parameters
-
[in] _isInsertMode True if the insert mode is activated [in] _cursorSize The sizae of the cursor that might be set when insert mode is set [default 20]
§ printDecorated() [1/2]
void ewol::compositing::TextBase::printDecorated | ( | const std::string & | _text | ) |
display a compleat string in the current element with the generic decoration specification. (basic html data)
[code style=xml]
an an other thext
<left> plop 1 </left>
<right> plop 2 </right>
<justify> Un exemple de text </justify> [/code]
- Note
- This is parsed with tiny xml, then be carfull that the XML is correct, and all balises are closed ... otherwite the display can not be done
- Parameters
-
[in] _text The string to display. : implementation not done ....
§ printDecorated() [2/2]
void ewol::compositing::TextBase::printDecorated | ( | const std::u32string & | _text | ) |
§ printHTML() [1/2]
void ewol::compositing::TextBase::printHTML | ( | const std::string & | _text | ) |
display a compleat string in the current element with the generic decoration specification. (basic html data)
[code style=xml] <html> <body>
an an other thext
<left> plop 1 </left>
<right> plop 2 </right>
<justify> Un exemple de text </justify> </body> </html> [/code]
- Note
- This is parsed with tiny xml, then be carfull that the XML is correct, and all balises are closed ... otherwite the display can not be done
- Parameters
-
[in] _text The string to display. : implementation not done ....
§ printHTML() [2/2]
void ewol::compositing::TextBase::printHTML | ( | const std::u32string & | _text | ) |
§ reset()
|
virtual |
clear all the intermediate result detween 2 prints
§ rotate()
|
virtual |
rotate the curent display of this element
- Parameters
-
[in] _vect The rotation vector to apply at the transformation matrix
Reimplemented from ewol::Compositing.
§ scale()
|
virtual |
scale the current diaplsy of this element
- Parameters
-
[in] _vect The scaling vector to apply at the transformation matrix
Reimplemented from ewol::Compositing.
§ setClipping() [1/2]
Request a clipping area for the text (next draw only)
- Parameters
-
[in] _pos Start position of the clipping [in] _posEnd End position of the clipping
§ setClipping() [2/2]
§ setClippingMode()
void ewol::compositing::TextBase::setClippingMode | ( | bool | _newMode | ) |
enable/Disable the clipping (without lose the current clipping position)
_newMode The new status of the clipping
§ setClippingWidth() [1/2]
|
inline |
Request a clipping area for the text (next draw only)
- Parameters
-
[in] _pos Start position of the clipping [in] _width Width size of the clipping
§ setClippingWidth() [2/2]
|
inline |
§ setColor()
|
inline |
set the Color of the current foreground font
- Parameters
-
[in] _color Color to set on foreground (for next print)
§ setColorBg()
void ewol::compositing::TextBase::setColorBg | ( | const etk::Color<> & | _color | ) |
set the background color of the font (for selected Text (not the global BG))
- Parameters
-
[in] _color Color to set on background (for next print)
§ setCursorColor()
void ewol::compositing::TextBase::setCursorColor | ( | const etk::Color<> & | _color | ) |
change the cursor color
- Parameters
-
[in] _color New color for the Selection
§ setCursorPos()
void ewol::compositing::TextBase::setCursorPos | ( | int32_t | _cursorPos | ) |
set a cursor at a specific position:
- Parameters
-
[in] _cursorPos id of the cursor position
§ setCursorSelection()
void ewol::compositing::TextBase::setCursorSelection | ( | int32_t | _cursorPos, |
int32_t | _selectionStartPos | ||
) |
set a cursor at a specific position with his associated selection:
- Parameters
-
[in] _cursorPos id of the cursor position [in] _selectionStartPos id of the starting of the selection
§ setDefaultColorBg()
|
inline |
set the default background color of the font (when reset, set this value ...)
- Parameters
-
[in] _color Color to set on background
§ setDefaultColorFg()
|
inline |
set the default Foreground color of the font (when reset, set this value ...)
- Parameters
-
[in] _color Color to set on foreground
§ setFont()
|
pure virtual |
Specify the font property (this reset the internal element of the current text (system requirement)
- Parameters
-
[in] fontName Current name of the selected font [in] fontSize New font size
Implemented in ewol::compositing::TextDF, and ewol::compositing::Text.
§ setFontBold()
void ewol::compositing::TextBase::setFontBold | ( | bool | _status | ) |
enable or disable the bold mode
- Parameters
-
[in] _status The new status for this display property
§ setFontItalic()
void ewol::compositing::TextBase::setFontItalic | ( | bool | _status | ) |
enable or disable the italic mode
- Parameters
-
[in] _status The new status for this display property
§ setFontMode()
|
pure virtual |
Specify the font mode for the next print.
- Parameters
-
[in] mode The font mode requested
Implemented in ewol::compositing::TextDF, and ewol::compositing::Text.
§ setFontName()
|
pure virtual |
Specify the font name (this reset the internal element of the current text (system requirement)
- Parameters
-
[in] _fontName Current name of the selected font
Implemented in ewol::compositing::TextDF, and ewol::compositing::Text.
§ setFontSize()
|
pure virtual |
Specify the font size (this reset the internal element of the current text (system requirement)
- Parameters
-
[in] _fontSize New font size
Implemented in ewol::compositing::TextDF, and ewol::compositing::Text.
§ setKerningMode()
void ewol::compositing::TextBase::setKerningMode | ( | bool | _newMode | ) |
set the activation of the Kerning for the display (if it existed)
- Parameters
-
[in] _newMode enable/Diasable the kerning on this font.
§ setPos() [1/2]
void ewol::compositing::TextBase::setPos | ( | const vec3 & | _pos | ) |
set position for the next text writen
- Parameters
-
[in] _pos Position of the text (in 3D)
§ setPos() [2/2]
|
inline |
§ setRelPos() [1/2]
void ewol::compositing::TextBase::setRelPos | ( | const vec3 & | _pos | ) |
set relative position for the next text writen
- Parameters
-
[in] _pos ofset apply of the text (in 3D)
§ setRelPos() [2/2]
|
inline |
§ setSelectionColor()
void ewol::compositing::TextBase::setSelectionColor | ( | const etk::Color<> & | _color | ) |
change the selection color
- Parameters
-
[in] _color New color for the Selection
§ setTextAlignement()
void ewol::compositing::TextBase::setTextAlignement | ( | float | _startTextpos, |
float | _stopTextPos, | ||
enum ewol::compositing::aligneMode | _alignement = ewol::compositing::alignDisable |
||
) |
This generate the possibility to generate the big text property.
- Parameters
-
[in] _startTextpos The x text start position of the display. [in] _stopTextPos The x text stop position of the display. [in] _alignement mode of alignement for the Text.
- Note
- The text align in center change of line every display done (even if it was just a char)
§ translate()
|
virtual |
translate the current display of this element
- Parameters
-
[in] _vect The translation vector to apply at the transformation matrix
Reimplemented from ewol::Compositing.
Member Data Documentation
§ m_alignement
|
protected |
Current Alignement mode (justify/left/right ...)
§ m_clippingEnable
|
protected |
true if the clipping must be activated
§ m_clippingPosStart
|
protected |
Clipping start position.
§ m_clippingPosStop
|
protected |
Clipping stop position.
§ m_color
|
protected |
The text foreground color.
§ m_colorBg
|
protected |
The text background color.
§ m_colorCursor
|
protected |
The text cursor color.
§ m_colorSelection
|
protected |
The text Selection color.
§ m_coord
|
protected |
internal coord of the object
§ m_coordColor
|
protected |
internal color of the different point
§ m_coordTex
|
protected |
internal texture coordinate for every point
§ m_cursorPos
|
protected |
Cursor position (default no cursor == > -100)
§ m_defaultColorBg
|
protected |
The text background color.
§ m_defaultColorFg
|
protected |
The text foreground color.
§ m_GLColor
|
protected |
openGL id on the element (color buffer)
§ m_GLMatrix
|
protected |
openGL id on the element (transformation matrix)
§ m_GLPosition
|
protected |
openGL id on the element (vertex buffer)
§ m_GLprogram
|
protected |
pointer on the opengl display program
§ m_GLtexID
|
protected |
openGL id on the element (texture ID)
§ m_GLtextHeight
|
protected |
openGL Id on the texture height
§ m_GLtexture
|
protected |
openGL id on the element (Texture position)
§ m_GLtextWidth
|
protected |
openGL Id on the texture width
§ m_htmlCurrrentLine
|
protected |
current line for HTML display
§ m_htmlDecoration
|
protected |
current decoration for the HTML display
§ m_htmlDecoTmp
|
protected |
current decoration
§ m_kerning
|
protected |
Kerning enable or disable on the next elements displayed.
§ m_mode
|
protected |
font display property : Regular/Bold/Italic/BoldItalic
§ m_nbCharDisplayed
|
protected |
prevent some error in calculation size.
§ m_needDisplay
|
protected |
This just need the display and not the size rendering.
§ m_position
|
protected |
The current position to draw.
§ m_previousCharcode
|
protected |
we remember the previous charcode to perform the kerning. Kerning
§ m_selectionStartPos
|
protected |
start position of the Selection (if == m_cursorPos ==> no selection)
§ m_sizeDisplayStart
|
protected |
The start windows of the display.
§ m_sizeDisplayStop
|
protected |
The end windows of the display.
§ m_startTextpos
|
protected |
start position of the Alignement (when
the text return at this position)
§ m_stopTextPos
|
protected |
end of the alignement (when a string is too hight it cut at the word previously this virtual line and the center is perform with this one)
§ m_vectorialDraw
|
protected |
This is used to draw background selection and other things ...
The documentation for this class was generated from the following file:
- framework/atria-soft/ewol/ewol/compositing/TextBase.hpp