#include <Shaper.hpp>
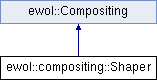
Public Member Functions | |
Shaper (const std::string &_shaperName="") | |
virtual | ~Shaper () |
void | draw (bool _disableDepthTest=true) |
void | clear () |
bool | setState (int32_t _newState) |
bool | changeStatusIn (int32_t _newStatusId) |
int32_t | getCurrentDisplayedStatus () |
int32_t | getNextDisplayedStatus () |
float | getTransitionStatus () |
bool | periodicCall (const ewol::event::Time &_event) |
ewol::Padding | getPadding () |
ewol::Padding | getPaddingIn () |
ewol::Padding | getPaddingOut () |
ewol::Padding | getBorder () |
void | setSource (const std::string &_newFile) |
const std::string & | getSource () const |
bool | hasSources () |
void | setShape (const vec2 &_origin, const vec2 &_size, const vec2 &_insidePos, const vec2 &_insideSize) |
void | setShape (const vec2 &_origin, const vec2 &_size) |
int32_t | requestColor (const std::string &_name) |
const etk::Color< float > & | getColor (int32_t _id) |
int32_t | requestConfig (const std::string &_name) |
double | getConfigNumber (int32_t _id) |
void | setActivateState (int32_t _status) |
bool | operator== (const Shaper &_obj) const |
bool | operator!= (const Shaper &_obj) const |
![]() | |
Compositing () | |
virtual | ~Compositing () |
virtual void | resetMatrix () |
virtual void | translate (const vec3 &_vect) |
virtual void | rotate (const vec3 &_vect, float _angle) |
virtual void | scale (const vec3 &_vect) |
virtual void | setMatrix (const mat4 &_mat) |
Additional Inherited Members | |
![]() | |
mat4 | m_matrixApply |
Detailed Description
the Shaper system is a basic theme configuration for every widget, it corespond at a background display described by a pool of files
Constructor & Destructor Documentation
§ Shaper()
ewol::compositing::Shaper::Shaper | ( | const std::string & | _shaperName = "" | ) |
generic constructor
- Parameters
-
[in] _shaperName Name of the file that might be loaded
§ ~Shaper()
|
virtual |
generic destructor
Member Function Documentation
§ changeStatusIn()
bool ewol::compositing::Shaper::changeStatusIn | ( | int32_t | _newStatusId | ) |
change the current status in an other
- Parameters
-
[in] _newStatusId the next new status requested
- Returns
- true The widget must call this fuction periodicly (and redraw itself)
- false No need to request the periodic call.
§ clear()
|
virtual |
clear alll tre registered element in the current element
Reimplemented from ewol::Compositing.
§ draw()
|
virtual |
draw All the refistered text in the current element on openGL
Implements ewol::Compositing.
§ getBorder()
ewol::Padding ewol::compositing::Shaper::getBorder | ( | ) |
get the padding declared by the user in the config file
- Returns
- the padding property
§ getColor()
const etk::Color<float>& ewol::compositing::Shaper::getColor | ( | int32_t | _id | ) |
Get The color associated at an ID.
- Parameters
-
[in] _id Id of the color
- Returns
- the reference on the color
§ getConfigNumber()
double ewol::compositing::Shaper::getConfigNumber | ( | int32_t | _id | ) |
Get The number associated at an ID.
- Parameters
-
[in] _id Id of the parameter
- Returns
- the requested number.
§ getCurrentDisplayedStatus()
|
inline |
get the current displayed status of the shaper
- Returns
- The Status Id
§ getNextDisplayedStatus()
|
inline |
get the next displayed status of the shaper
- Returns
- The next status Id (-1 if no status in next)
§ getPadding()
ewol::Padding ewol::compositing::Shaper::getPadding | ( | ) |
get the padding declared by the user in the config file
- Returns
- the padding property
§ getSource()
|
inline |
get the shaper file Source
- Returns
- the shapper file name
§ getTransitionStatus()
|
inline |
get the current trasion status
- Returns
- value of the transition status (0.0f when no activity)
§ hasSources()
bool ewol::compositing::Shaper::hasSources | ( | ) |
Sometimes the user declare an image but not allocate the ressources all the time, this is to know it ..
- Returns
- the validity od the resources.
§ periodicCall()
bool ewol::compositing::Shaper::periodicCall | ( | const ewol::event::Time & | _event | ) |
Same as the widfget periodic call (this is for change display)
- Parameters
-
[in] _event The current time of the call.
- Returns
- true The widget must call this fuction periodicly (and redraw itself)
- false No need to request the periodic call.
§ requestColor()
int32_t ewol::compositing::Shaper::requestColor | ( | const std::string & | _name | ) |
Get an ID on the color instance element.
- Parameters
-
[in] _name Name of the element requested
- Returns
- The Id of the color
§ requestConfig()
int32_t ewol::compositing::Shaper::requestConfig | ( | const std::string & | _name | ) |
Get an ID on the configuration instance element.
- Parameters
-
[in] _name Name of the element requested
- Returns
- The Id of the element
§ setActivateState()
|
inline |
Set activate state of the element.
- Parameters
-
[in] _status New activate status
§ setShape()
void ewol::compositing::Shaper::setShape | ( | const vec2 & | _origin, |
const vec2 & | _size, | ||
const vec2 & | _insidePos, | ||
const vec2 & | _insideSize | ||
) |
set the shape property:
- _size *
- *
- * - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - * *
- *
- | | *
- | * * | *
- | * * - - - - - - - - - - - - - - - - - - * * | *
- * _insideSize * *
- | * | | * | *
- | * | | * | *
- | * | | * | *
- | * | | * | *
- | * | | * | *
- | * | | * | *
- * _insidePos * *
- | * * - - - - - - - - - - - - - - - - - - * * | *
- | *************************************************** | *
- *
- | | *
- *
- * - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - * *
- *
- *
_origin
- Parameters
-
[in] _origin Origin of the display [in] _size Size of the display [in] _insidePos Positin of the internal data [in] _insideSize Size of the internal data
§ setSource()
void ewol::compositing::Shaper::setSource | ( | const std::string & | _newFile | ) |
change the shaper Source
- Parameters
-
[in] _newFile New file of the shaper
§ setState()
bool ewol::compositing::Shaper::setState | ( | int32_t | _newState | ) |
Change the current state.
- Parameters
-
[in] _newState Current state of the configuration
- Returns
- true Need redraw.
- false No need redraw.
The documentation for this class was generated from the following file:
- framework/atria-soft/ewol/ewol/compositing/Shaper.hpp