#include <PropertyType.hpp>
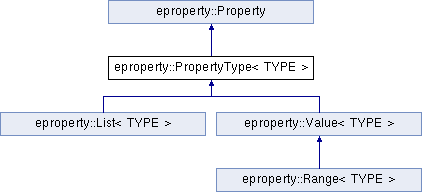
Public Member Functions | |
template<class CLASS_TYPE > | |
PropertyType (CLASS_TYPE *_owner, const std::string &_name, const TYPE &_defaultValue, const std::string &_description="", void(CLASS_TYPE::*_setObs)()=nullptr) | |
PropertyType (const TYPE &_defaultValue) | |
virtual | ~PropertyType ()=default |
std::string | getPropertyType () const override |
std::string | getType () const override |
std::string | getString () const override |
std::string | getDefault () const override |
std::string | getInfo () const override |
bool | isDefault () const override |
void | setDefault () override |
virtual void | changeDefault (const TYPE &_newDefault) |
const TYPE & | get () const |
virtual void | set (const TYPE &_newVal) |
void | setDirect (const TYPE &_newVal) |
virtual void | setDirectCheck (const TYPE &_newVal) |
TYPE & | getDirect () |
virtual std::string | getValueSpecific (const TYPE &_valueRequested) const =0 |
operator const TYPE & () const | |
const TYPE & | operator* () const noexcept |
const TYPE * | operator-> () const noexcept |
PropertyType< TYPE > & | operator= (const TYPE &_newVal)=delete |
![]() | |
Property (eproperty::Interface *_paramInterfaceLink, const std::string &_name) | |
Property () | |
virtual | ~Property ()=default |
void | notifyChange () const |
virtual std::string | getName () const |
virtual void | setString (const std::string &_newVal)=0 |
virtual std::vector< std::string > | getListValue () const |
template<class TYPE > | |
bool | operator== (const TYPE &_obj) const =delete |
template<class TYPE > | |
bool | operator!= (const TYPE &_obj) const =delete |
template<class TYPE > | |
bool | operator<= (const TYPE &_obj) const =delete |
template<class TYPE > | |
bool | operator>= (const TYPE &_obj) const =delete |
template<class TYPE > | |
bool | operator< (const TYPE &_obj) const =delete |
template<class TYPE > | |
bool | operator> (const TYPE &_obj) const =delete |
Protected Attributes | |
TYPE | m_value |
TYPE | m_default |
Additional Inherited Members | |
![]() | |
using | Observer = std::function< void()> |
![]() | |
void | setObserver (eproperty::Property::Observer _setObs) |
Detailed Description
template<class TYPE>
class eproperty::PropertyType< TYPE >
Template base of the property (have a generic set and get for string)
Constructor & Destructor Documentation
§ PropertyType() [1/2]
|
inline |
Create a parameter with a specific type.
- Parameters
-
[in] _owner Owner of the parameter (nullptr if none). [in] _name Static name of the parameter. [in] _defaultValue Default value of the parameter. [in] _description description of the parameter. [in] _setObs function of the class that opserve the change of the value
§ PropertyType() [2/2]
|
inline |
Create a parameter with a specific type.
- Parameters
-
[in] _defaultValue Default value of the parameter.
§ ~PropertyType()
|
virtualdefault |
Destructor.
Member Function Documentation
§ changeDefault()
|
inlinevirtual |
Set new default value on the property.
- Parameters
-
[in] _newDefault New value to set
§ get()
|
inline |
Get the value of the current parameter.
- Note
- For performence, this function must be inline
- Returns
- the Reference value
§ getDefault()
|
inlineoverridevirtual |
Get the string of the default value of the Property.
- Returns
- the string decription of the default value.
Implements eproperty::Property.
§ getDirect()
|
inline |
Get the value of the current parameter (no check (for internal set with no check).
- Note
- For performence, this function must be inline
- Only use by the owner of the property (can not be check on compile time for now ...) TODO: Do it better ... compile check
- Returns
- a reference on the value
§ getInfo()
|
inlineoverridevirtual |
Description of the Propertys.
- Returns
- Descriptive information of the Property (for remote UI).
Implements eproperty::Property.
Reimplemented in eproperty::Range< TYPE >.
§ getPropertyType()
|
inlineoverridevirtual |
Get the Property type of the class in string mode.
- Returns
- The string type of the Property.
Implements eproperty::Property.
Reimplemented in eproperty::Range< TYPE >.
§ getString()
|
inlineoverridevirtual |
Get the string of the current value of the Property.
- Returns
- The string description of the value.
Implements eproperty::Property.
§ getType()
|
inlineoverridevirtual |
Get the type of the Property in string mode.
- Returns
- The string type of the Property.
Implements eproperty::Property.
§ getValueSpecific()
|
pure virtual |
Get the string of the specify value.
- Parameters
-
[in] _valueRequested Value to convert in string
- Returns
- convertion of the value in string.
Implemented in eproperty::Value< TYPE >.
§ isDefault()
|
inlineoverridevirtual |
Check if the value is the default.
- Returns
- true : the vakue is the default one, false otherwise.
Implements eproperty::Property.
§ operator const TYPE &()
|
inline |
Const cast the property in the Type of the data.
- Returns
- Const reference on the value.
§ operator*()
|
inlinenoexcept |
Get the property Value.
- Returns
- Const reference on the value.
§ operator->()
|
inlinenoexcept |
Get the property Value.
- Returns
- Const reference on the value.
§ operator=()
|
delete |
§ set()
|
inlinevirtual |
Set a new value for this parameter.
- Parameters
-
[in] _newVal New value to set (set the nearest value if range is set)
Reimplemented in eproperty::List< TYPE >, and eproperty::Range< TYPE >.
§ setDefault()
|
inlineoverridevirtual |
Reset the value to the default value.
Implements eproperty::Property.
§ setDirect()
|
inline |
Set the value of the current parameter (no check (for internal set with no check).
- Note
- For performence, this function must be inline
- Only use by the owner of the property (can not be check on compile time for now ...) TODO: Do it better ... compile check
- Parameters
-
[in] _newVal New value to set
§ setDirectCheck()
|
inlinevirtual |
Set the value of the current parameter (check range and ... if needed).
- Note
- Only use by the owner of the property/
- Parameters
-
[in] _newVal New value to set
Reimplemented in eproperty::List< TYPE >, and eproperty::Range< TYPE >.
Member Data Documentation
§ m_default
|
protected |
Default value.
§ m_value
|
protected |
Current value.
The documentation for this class was generated from the following file:
- framework/atria-soft/eproperty/eproperty/PropertyType.hpp