#include <Property.hpp>
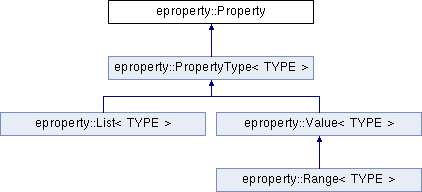
Public Types | |
using | Observer = std::function< void()> |
Public Member Functions | |
Property (eproperty::Interface *_paramInterfaceLink, const std::string &_name) | |
Property () | |
virtual | ~Property ()=default |
void | notifyChange () const |
virtual std::string | getName () const |
virtual std::string | getInfo () const =0 |
virtual std::string | getPropertyType () const =0 |
virtual std::string | getType () const =0 |
virtual std::string | getString () const =0 |
virtual std::string | getDefault () const =0 |
virtual void | setString (const std::string &_newVal)=0 |
virtual bool | isDefault () const =0 |
virtual void | setDefault ()=0 |
virtual std::vector< std::string > | getListValue () const |
template<class TYPE > | |
bool | operator== (const TYPE &_obj) const =delete |
template<class TYPE > | |
bool | operator!= (const TYPE &_obj) const =delete |
template<class TYPE > | |
bool | operator<= (const TYPE &_obj) const =delete |
template<class TYPE > | |
bool | operator>= (const TYPE &_obj) const =delete |
template<class TYPE > | |
bool | operator< (const TYPE &_obj) const =delete |
template<class TYPE > | |
bool | operator> (const TYPE &_obj) const =delete |
Protected Member Functions | |
void | setObserver (eproperty::Property::Observer _setObs) |
Detailed Description
Base of the property With all generic element needed.
Member Typedef Documentation
§ Observer
using eproperty::Property::Observer = std::function<void()> |
Local main object observer of changing value of the property.
Constructor & Destructor Documentation
§ Property() [1/2]
eproperty::Property::Property | ( | eproperty::Interface * | _paramInterfaceLink, |
const std::string & | _name | ||
) |
Basic property elements.
- Parameters
-
[in] _paramInterfaceLink Link on the esignal::Interface class to register parameter (can be nullptr) [in] _name Name of the parameter (must be unique if _paramInterfaceLink is define)
§ Property() [2/2]
eproperty::Property::Property | ( | ) |
Basic property elements.
§ ~Property()
|
virtualdefault |
Virtualize the destructor.
Member Function Documentation
§ getDefault()
|
pure virtual |
Get the string of the default value of the Property.
- Returns
- the string decription of the default value.
Implemented in eproperty::PropertyType< TYPE >.
§ getInfo()
|
pure virtual |
Description of the Propertys.
- Returns
- Descriptive information of the Property (for remote UI).
Implemented in eproperty::List< TYPE >, eproperty::PropertyType< TYPE >, and eproperty::Range< TYPE >.
§ getListValue()
|
inlinevirtual |
Specific for eproperty::List to get all the possible values.
- Returns
- Descriptive information of the Property (for remote UI).
Reimplemented in eproperty::List< TYPE >.
§ getName()
|
virtual |
§ getPropertyType()
|
pure virtual |
Get the Property type of the class in string mode.
- Returns
- The string type of the Property.
Implemented in eproperty::List< TYPE >, eproperty::Range< TYPE >, and eproperty::PropertyType< TYPE >.
§ getString()
|
pure virtual |
Get the string of the current value of the Property.
- Returns
- The string description of the value.
Implemented in eproperty::PropertyType< TYPE >.
§ getType()
|
pure virtual |
Get the type of the Property in string mode.
- Returns
- The string type of the Property.
Implemented in eproperty::PropertyType< TYPE >.
§ isDefault()
|
pure virtual |
Check if the value is the default.
- Returns
- true : the vakue is the default one, false otherwise.
Implemented in eproperty::PropertyType< TYPE >.
§ notifyChange()
void eproperty::Property::notifyChange | ( | ) | const |
call main class that PropertyChange
§ operator!=()
|
delete |
IN-Eguality comparaison operator (REMOVED)
- Parameters
-
[in] _obj Object to compare
- Returns
- true The current object is NOT identic
- false The current object is identic
§ operator<()
|
delete |
Lesser comparaison operator (REMOVED)
- Parameters
-
[in] _obj Object to compare
- Returns
- true The current object lesser than input object
- false The current object greater or equal than input object
§ operator<=()
|
delete |
Lesser eguality comparaison operator (REMOVED)
- Parameters
-
[in] _obj Object to compare
- Returns
- true The current object lesser or equal than input object
- false The current object greater than input object
§ operator==()
|
delete |
Eguality comparaison operator (REMOVED)
- Parameters
-
[in] _obj Object to compare
- Returns
- true The current object is identic
- false The current object is NOT identic
§ operator>()
|
delete |
Greater comparaison operator (REMOVED)
- Parameters
-
[in] _obj Object to compare
- Returns
- true The current object greater than input object
- false The current object lesser or equal than input object
§ operator>=()
|
delete |
Greater eguality comparaison operator (REMOVED)
- Parameters
-
[in] _obj Object to compare
- Returns
- true The current object greater or equal than input object
- false The current object lesser than input object
§ setDefault()
|
pure virtual |
Reset the value to the default value.
Implemented in eproperty::PropertyType< TYPE >.
§ setObserver()
|
protected |
Set the change observer of the property.
- Parameters
-
[in] _setObs New observer of the property
§ setString()
|
pure virtual |
Set a new value of the Property (with string interface).
- Parameters
-
[in] _newVal New value of the Propertys.
Implemented in eproperty::List< TYPE >, eproperty::Range< TYPE >, and eproperty::Value< TYPE >.
The documentation for this class was generated from the following file:
- framework/atria-soft/eproperty/eproperty/Property.hpp