#include <String.hpp>
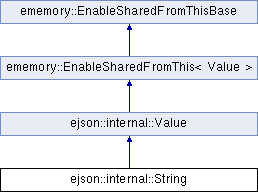
Public Member Functions | |
void | set (const std::string &_value) |
const std::string & | get () const |
bool | iParse (const std::string &_data, size_t &_pos, ejson::FilePos &_filePos, ejson::internal::Document &_doc) override |
bool | iGenerate (std::string &_data, size_t _indent) const override |
void | iMachineGenerate (std::string &_data) const override |
bool | transfertIn (ememory::SharedPtr< ejson::internal::Value > _obj) override |
ememory::SharedPtr< ejson::internal::Value > | clone () const override |
![]() | |
enum ejson::valueType | getType () const |
virtual | ~Value () |
void | display () const |
virtual void | clear () |
![]() | |
ememory::SharedPtr< EMEMORY_TYPE > | sharedFromThis () |
const ememory::SharedPtr< EMEMORY_TYPE > | sharedFromThis () const |
ememory::WeakPtr< EMEMORY_TYPE > | weakFromThis () |
const ememory::WeakPtr< EMEMORY_TYPE > | weakFromThis () const |
Static Public Member Functions | |
static ememory::SharedPtr< String > | create (const std::string &_value="") |
Protected Member Functions | |
String (const std::string &_value="") | |
![]() | |
Value () | |
void | addIndent (std::string &_data, int32_t _indent) const |
void | drawElementParsed (char32_t _val, const ejson::FilePos &_filePos) const |
bool | checkString (char32_t _val) const |
bool | checkNumber (char32_t _val) const |
int32_t | countWhiteChar (const std::string &_data, size_t _pos, ejson::FilePos &_filePos) const |
Protected Attributes | |
std::string | m_value |
![]() | |
enum ejson::valueType | m_type |
Additional Inherited Members | |
![]() | |
static bool | isWhiteChar (char32_t _val) |
Detailed Description
ejson String internal data implementation.
Constructor & Destructor Documentation
§ String()
|
protected |
basic element of a xml structure
- Parameters
-
[in] _value Value to set on the ejson::Value
Member Function Documentation
§ clone()
|
overridevirtual |
Copy the curent node and all the child in the curent one.
- Returns
- nullptr in an error occured, the pointer on the element otherwise
Reimplemented from ejson::internal::Value.
§ create()
|
static |
Create factory on the ejson::internal::String.
- Parameters
-
[in] _value Value to set on the ejson::Value
- Returns
- A SharedPtr on the String value
§ get()
const std::string& ejson::internal::String::get | ( | ) | const |
get the current element Value.
- Returns
- the reference of the string value.
§ iGenerate()
|
overridevirtual |
generate a string with the tree of the json
- Parameters
-
[in,out] _data string where to add the elements [in] _indent current indentation of the file
- Returns
- false if an error occured.
Implements ejson::internal::Value.
§ iMachineGenerate()
|
overridevirtual |
generate a string with the tree of the json (not human readable ==> for computer transfer)
- Parameters
-
[in,out] _data string where to add the elements
- Returns
- false if an error occured.
Implements ejson::internal::Value.
§ iParse()
|
overridevirtual |
parse the Current node [pure VIRUAL]
- Parameters
-
[in] _data data string to parse. [in,out] _pos position in the string to start parse, return the position end of parsing. [in,out] _filePos Position in the file (in X/Y) [in,out] _doc Reference on the main document
- Returns
- false if an error occured.
Implements ejson::internal::Value.
§ set()
void ejson::internal::String::set | ( | const std::string & | _value | ) |
set the value of the node.
- Parameters
-
[in] _value New value of the node.
§ transfertIn()
|
overridevirtual |
Tranfert all element in the element set in parameter.
- Parameters
-
[in,out] _obj move all parameter in the selected element
- Returns
- true if transfer is done corectly
- Note
- all element is remove from the curent element.
Reimplemented from ejson::internal::Value.
Member Data Documentation
§ m_value
|
protected |
value of the node (for element this is the name, for text it is the inside text ...)
The documentation for this class was generated from the following file:
- framework/atria-soft/ejson/ejson/internal/String.hpp