ejson::internal::Document Class Reference
#include <Document.hpp>
Inheritance diagram for ejson::internal::Document:
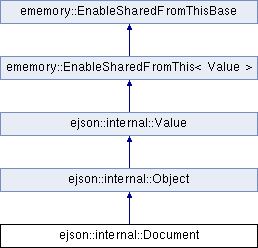
Public Member Functions | |
Document () | |
bool | parse (const std::string &_data) |
bool | generate (std::string &_data) |
bool | load (const std::string &_file) |
bool | store (const std::string &_file) |
void | setDisplayError (bool _value) |
bool | getDisplayError () |
void | displayError () |
void | createError (const std::string &_data, size_t _pos, const ejson::FilePos &_filePos, const std::string &_comment) |
bool | iParse (const std::string &_data, size_t &_pos, ejson::FilePos &_filePos, ejson::internal::Document &_doc) override |
bool | iGenerate (std::string &_data, size_t _indent) const override |
![]() | |
bool | exist (const std::string &_name) const |
ememory::SharedPtr< ejson::internal::Value > | get (const std::string &_name) |
const ememory::SharedPtr< ejson::internal::Value > | get (const std::string &_name) const |
std::vector< std::string > | getKeys () const |
size_t | size () const |
ememory::SharedPtr< ejson::internal::Value > | get (size_t _id) |
const ememory::SharedPtr< ejson::internal::Value > | get (size_t _id) const |
std::string | getKey (size_t _id) const |
bool | add (const std::string &_name, ememory::SharedPtr< ejson::internal::Value > _value) |
void | remove (const std::string &_name) |
void | remove (size_t _id) |
bool | cloneIn (ememory::SharedPtr< ejson::internal::Object > &_obj) const |
ememory::SharedPtr< ejson::internal::Object > | cloneObj () const |
bool | iParse (const std::string &_data, size_t &_pos, ejson::FilePos &_filePos, ejson::internal::Document &_doc) override |
bool | iGenerate (std::string &_data, size_t _indent) const override |
void | iMachineGenerate (std::string &_data) const override |
void | clear () override |
bool | transfertIn (ememory::SharedPtr< ejson::internal::Value > _obj) override |
ememory::SharedPtr< ejson::internal::Value > | clone () const override |
![]() | |
enum ejson::valueType | getType () const |
virtual | ~Value () |
void | display () const |
![]() | |
ememory::SharedPtr< EMEMORY_TYPE > | sharedFromThis () |
const ememory::SharedPtr< EMEMORY_TYPE > | sharedFromThis () const |
ememory::WeakPtr< EMEMORY_TYPE > | weakFromThis () |
const ememory::WeakPtr< EMEMORY_TYPE > | weakFromThis () const |
Static Public Member Functions | |
static ememory::SharedPtr< Document > | create () |
![]() | |
static ememory::SharedPtr< Object > | create () |
static ememory::SharedPtr< Object > | create (const std::string &_data) |
Additional Inherited Members | |
![]() | |
Object () | |
![]() | |
Value () | |
void | addIndent (std::string &_data, int32_t _indent) const |
void | drawElementParsed (char32_t _val, const ejson::FilePos &_filePos) const |
bool | checkString (char32_t _val) const |
bool | checkNumber (char32_t _val) const |
int32_t | countWhiteChar (const std::string &_data, size_t _pos, ejson::FilePos &_filePos) const |
![]() | |
static bool | isWhiteChar (char32_t _val) |
![]() | |
etk::Hash< ememory::SharedPtr< ejson::internal::Value > > | m_value |
![]() | |
enum ejson::valueType | m_type |
Detailed Description
ejson Document internal data implementation.
Constructor & Destructor Documentation
§ Document()
ejson::internal::Document::Document | ( | ) |
Constructor.
Member Function Documentation
§ create()
|
static |
Create factory on the ejson::internal::Document.
- Returns
- A SharedPtr on the Document value
§ createError()
void ejson::internal::Document::createError | ( | const std::string & | _data, |
size_t | _pos, | ||
const ejson::FilePos & | _filePos, | ||
const std::string & | _comment | ||
) |
When parsing a subParser create an error that might be write later.
- Parameters
-
[in] _data Wall File or stream [in] _pos Position in the file (in nb char) [in] _filePos Position in x/y in the file [in] _comment Help coment
§ displayError()
void ejson::internal::Document::displayError | ( | ) |
Display error detected.
§ generate()
bool ejson::internal::Document::generate | ( | std::string & | _data | ) |
generate a string that contain the created XML
- Parameters
-
[out] _data Data where the xml is stored
- Returns
- false : An error occured
- true : Parsing is OK
§ getDisplayError()
bool ejson::internal::Document::getDisplayError | ( | ) |
Get the display of the error status.
- Returns
- true Display error
- false Does not display error (get it at end)
§ iGenerate()
|
overridevirtual |
generate a string with the tree of the json
- Parameters
-
[in,out] _data string where to add the elements [in] _indent current indentation of the file
- Returns
- false if an error occured.
Implements ejson::internal::Value.
§ iParse()
|
overridevirtual |
parse the Current node [pure VIRUAL]
- Parameters
-
[in] _data data string to parse. [in,out] _pos position in the string to start parse, return the position end of parsing. [in,out] _filePos Position in the file (in X/Y) [in,out] _doc Reference on the main document
- Returns
- false if an error occured.
Implements ejson::internal::Value.
§ load()
bool ejson::internal::Document::load | ( | const std::string & | _file | ) |
Load the file that might contain the xml.
- Parameters
-
[in] _file Filename of the xml (compatible with etk FSNode naming)
- Returns
- false : An error occured
- true : Parsing is OK
§ parse()
bool ejson::internal::Document::parse | ( | const std::string & | _data | ) |
parse a string that contain an XML
- Parameters
-
[in] _data Data to parse
- Returns
- false : An error occured
- true : Parsing is OK
§ setDisplayError()
void ejson::internal::Document::setDisplayError | ( | bool | _value | ) |
Set the display of the error when detected.
- Parameters
-
[in] _value true: display error, false not display error (get it at end)
§ store()
bool ejson::internal::Document::store | ( | const std::string & | _file | ) |
Store the Xml in the file.
- Parameters
-
[in] _file Filename of the xml (compatible with etk FSNode naming)
- Returns
- false : An error occured
- true : Parsing is OK
The documentation for this class was generated from the following file:
- framework/atria-soft/ejson/ejson/internal/Document.hpp