#include <Value.hpp>
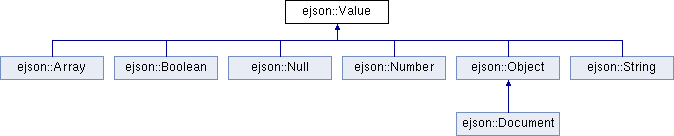
Public Member Functions | |
enum ejson::valueType | getType () const |
Value (const ememory::SharedPtr< ejson::internal::Value > &_internalValue) | |
Value () | |
virtual | ~Value ()=default |
void | display () const |
bool | exist () const |
ejson::Document | toDocument () |
const ejson::Document | toDocument () const |
ejson::Array | toArray () |
const ejson::Array | toArray () const |
ejson::Object | toObject () |
const ejson::Object | toObject () const |
ejson::String | toString () |
const ejson::String | toString () const |
ejson::Number | toNumber () |
const ejson::Number | toNumber () const |
ejson::Boolean | toBoolean () |
const ejson::Boolean | toBoolean () const |
ejson::Null | toNull () |
const ejson::Null | toNull () const |
bool | isDocument () const |
bool | isArray () const |
bool | isObject () const |
bool | isString () const |
bool | isNumber () const |
bool | isBoolean () const |
bool | isNull () const |
void | clear () |
bool | transfertIn (ejson::Value &_obj) |
ejson::Value | clone () const |
std::string | generateHumanString () const |
std::string | generateMachineString () const |
Protected Attributes | |
ememory::SharedPtr< ejson::internal::Value > | m_data |
Friends | |
class | ejson::Array |
class | ejson::Object |
Detailed Description
Basic main object of all json elements.
Constructor & Destructor Documentation
§ Value() [1/2]
ejson::Value::Value | ( | const ememory::SharedPtr< ejson::internal::Value > & | _internalValue | ) |
basic element of a xml structure
- Parameters
-
[in] _internalValue Internal reference of the Value.
§ Value() [2/2]
ejson::Value::Value | ( | ) |
basic element of a xml structure
§ ~Value()
|
virtualdefault |
Virtualize destructor.
Member Function Documentation
§ clear()
void ejson::Value::clear | ( | ) |
clear the Node
§ clone()
ejson::Value ejson::Value::clone | ( | ) | const |
Copy the curent node and all the child in the curent one.
- Returns
- nullptr in an error occured, the pointer on the element otherwise
§ display()
void ejson::Value::display | ( | ) | const |
Display the Document on console.
§ exist()
bool ejson::Value::exist | ( | ) | const |
Check if the element exit.
- Returns
- true The element exist
- False The element does NOT exist
§ generateHumanString()
std::string ejson::Value::generateHumanString | ( | ) | const |
generate a string that contain the created JSON
- Returns
- generated data
§ generateMachineString()
std::string ejson::Value::generateMachineString | ( | ) | const |
generate a string that contain the created JSON
- Returns
- generated data
§ getType()
enum ejson::valueType ejson::Value::getType | ( | ) | const |
Get Value type.
- Returns
- Type of the object
§ isArray()
bool ejson::Value::isArray | ( | ) | const |
check if the node is a ejson::Array
- Returns
- true if the node is a ejson::Array
§ isBoolean()
bool ejson::Value::isBoolean | ( | ) | const |
check if the node is a ejson::Boolean
- Returns
- true if the node is a ejson::Boolean
§ isDocument()
bool ejson::Value::isDocument | ( | ) | const |
check if the node is a ejson::Document
- Returns
- true if the node is a ejson::Document
§ isNull()
bool ejson::Value::isNull | ( | ) | const |
check if the node is a ejson::Null
- Returns
- true if the node is a ejson::Null
§ isNumber()
bool ejson::Value::isNumber | ( | ) | const |
check if the node is a ejson::Number
- Returns
- true if the node is a ejson::Number
§ isObject()
bool ejson::Value::isObject | ( | ) | const |
check if the node is a ejson::Object
- Returns
- true if the node is a ejson::Object
§ isString()
bool ejson::Value::isString | ( | ) | const |
check if the node is a ejson::String
- Returns
- true if the node is a ejson::String
§ toArray() [1/2]
ejson::Array ejson::Value::toArray | ( | ) |
Cast the element in a Array if it is possible.
- Returns
- pointer on the class or nullptr.
§ toArray() [2/2]
const ejson::Array ejson::Value::toArray | ( | ) | const |
Cast the element in a Array if it is possible.
- Returns
- CONST pointer on the class or nullptr.
§ toBoolean() [1/2]
ejson::Boolean ejson::Value::toBoolean | ( | ) |
Cast the element in a Boolean if it is possible.
- Returns
- pointer on the class or nullptr.
§ toBoolean() [2/2]
const ejson::Boolean ejson::Value::toBoolean | ( | ) | const |
Cast the element in a Boolean if it is possible.
- Returns
- CONST pointer on the class or nullptr.
§ toDocument() [1/2]
ejson::Document ejson::Value::toDocument | ( | ) |
Cast the element in a Document if it is possible.
- Returns
- pointer on the class or nullptr.
§ toDocument() [2/2]
const ejson::Document ejson::Value::toDocument | ( | ) | const |
Cast the element in a Document if it is possible.
- Returns
- CONST pointer on the class or nullptr.
§ toNull() [1/2]
ejson::Null ejson::Value::toNull | ( | ) |
Cast the element in a Null if it is possible.
- Returns
- pointer on the class or nullptr.
§ toNull() [2/2]
const ejson::Null ejson::Value::toNull | ( | ) | const |
Cast the element in a Null if it is possible.
- Returns
- CONST pointer on the class or nullptr.
§ toNumber() [1/2]
ejson::Number ejson::Value::toNumber | ( | ) |
Cast the element in a Number if it is possible.
- Returns
- pointer on the class or nullptr.
§ toNumber() [2/2]
const ejson::Number ejson::Value::toNumber | ( | ) | const |
Cast the element in a Number if it is possible.
- Returns
- CONST pointer on the class or nullptr.
§ toObject() [1/2]
ejson::Object ejson::Value::toObject | ( | ) |
Cast the element in a Object if it is possible.
- Returns
- pointer on the class or nullptr.
§ toObject() [2/2]
const ejson::Object ejson::Value::toObject | ( | ) | const |
Cast the element in a Object if it is possible.
- Returns
- CONST pointer on the class or nullptr.
§ toString() [1/2]
ejson::String ejson::Value::toString | ( | ) |
Cast the element in a String if it is possible.
- Returns
- pointer on the class or nullptr.
§ toString() [2/2]
const ejson::String ejson::Value::toString | ( | ) | const |
Cast the element in a String if it is possible.
- Returns
- CONST pointer on the class or nullptr.
§ transfertIn()
bool ejson::Value::transfertIn | ( | ejson::Value & | _obj | ) |
Tranfert all element in the element set in parameter.
- Parameters
-
[in,out] _obj move all parameter in the selected element
- Returns
- true if transfer is done corectly
- Note
- all element is remove from the curent element.
Member Data Documentation
§ m_data
|
protected |
internal reference on a Value
The documentation for this class was generated from the following file:
- framework/atria-soft/ejson/ejson/Value.hpp