#include <Object.hpp>
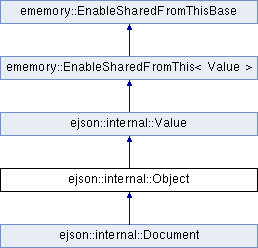
Static Public Member Functions | |
static ememory::SharedPtr< Object > | create () |
static ememory::SharedPtr< Object > | create (const std::string &_data) |
Protected Member Functions | |
Object () | |
![]() | |
Value () | |
void | addIndent (std::string &_data, int32_t _indent) const |
void | drawElementParsed (char32_t _val, const ejson::FilePos &_filePos) const |
bool | checkString (char32_t _val) const |
bool | checkNumber (char32_t _val) const |
int32_t | countWhiteChar (const std::string &_data, size_t _pos, ejson::FilePos &_filePos) const |
Protected Attributes | |
etk::Hash< ememory::SharedPtr< ejson::internal::Value > > | m_value |
![]() | |
enum ejson::valueType | m_type |
Additional Inherited Members | |
![]() | |
static bool | isWhiteChar (char32_t _val) |
Detailed Description
ejson Object internal data implementation.
Constructor & Destructor Documentation
§ Object()
|
inlineprotected |
basic element of a xml structure
Member Function Documentation
§ add()
bool ejson::internal::Object::add | ( | const std::string & | _name, |
ememory::SharedPtr< ejson::internal::Value > | _value | ||
) |
add an element in the Object
- Parameters
-
[in] _name name of the object [in] _value Element to add
- Returns
- false if an error occured
§ clear()
|
overridevirtual |
clear the Node
Reimplemented from ejson::internal::Value.
§ clone()
|
overridevirtual |
Copy the curent node and all the child in the curent one.
- Returns
- nullptr in an error occured, the pointer on the element otherwise
Reimplemented from ejson::internal::Value.
§ cloneIn()
bool ejson::internal::Object::cloneIn | ( | ememory::SharedPtr< ejson::internal::Object > & | _obj | ) | const |
Clone the current object in an other Object.
- Parameters
-
[in] _obj Other object ot overwride
- Returns
- true The clone has been corectly done, false otherwise
§ cloneObj()
ememory::SharedPtr<ejson::internal::Object> ejson::internal::Object::cloneObj | ( | ) | const |
Clone the current object.
- Returns
- A new object that has been clone
§ create() [1/2]
|
static |
Create factory on the ejson::internal::Object.
- Returns
- A SharedPtr on the Object value
§ create() [2/2]
|
static |
Create factory on the ejson::internal::Object.
- Parameters
-
[in] _data Json stream to parse and interprete
- Returns
- A SharedPtr on the Object value
§ exist()
bool ejson::internal::Object::exist | ( | const std::string & | _name | ) | const |
check if an element exist.
- Parameters
-
[in] _name name of the object.
- Returns
- The existance of the element.
§ get() [1/4]
ememory::SharedPtr<ejson::internal::Value> ejson::internal::Object::get | ( | const std::string & | _name | ) |
get the sub element with his name (no cast check)
- Parameters
-
[in] _name name of the object
- Returns
- pointer on the element requested or nullptr if it not the corect type or does not existed
§ get() [2/4]
const ememory::SharedPtr<ejson::internal::Value> ejson::internal::Object::get | ( | const std::string & | _name | ) | const |
get the sub element with his name (no cast check)
- Parameters
-
[in] _name name of the object
- Returns
- pointer on the element requested or nullptr if it not the corect type or does not existed
§ get() [3/4]
ememory::SharedPtr<ejson::internal::Value> ejson::internal::Object::get | ( | size_t | _id | ) |
get the pointer on an element reference with his ID.
- Parameters
-
[in] _id Id of the element.
- Returns
- nullptr if the element does not exist.
§ get() [4/4]
const ememory::SharedPtr<ejson::internal::Value> ejson::internal::Object::get | ( | size_t | _id | ) | const |
get the pointer on an element reference with his ID.
- Parameters
-
[in] _id Id of the element.
- Returns
- nullptr if the element does not exist.
§ getKey()
std::string ejson::internal::Object::getKey | ( | size_t | _id | ) | const |
Get the element name (key).
- Parameters
-
[in] _id Id of the element.
- Returns
- The name (key).
§ getKeys()
std::vector<std::string> ejson::internal::Object::getKeys | ( | ) | const |
Get all the element name (keys).
- Returns
- a vector of all name (key).
§ iGenerate()
|
overridevirtual |
generate a string with the tree of the json
- Parameters
-
[in,out] _data string where to add the elements [in] _indent current indentation of the file
- Returns
- false if an error occured.
Implements ejson::internal::Value.
§ iMachineGenerate()
|
overridevirtual |
generate a string with the tree of the json (not human readable ==> for computer transfer)
- Parameters
-
[in,out] _data string where to add the elements
- Returns
- false if an error occured.
Implements ejson::internal::Value.
§ iParse()
|
overridevirtual |
parse the Current node [pure VIRUAL]
- Parameters
-
[in] _data data string to parse. [in,out] _pos position in the string to start parse, return the position end of parsing. [in,out] _filePos Position in the file (in X/Y) [in,out] _doc Reference on the main document
- Returns
- false if an error occured.
Implements ejson::internal::Value.
§ remove() [1/2]
void ejson::internal::Object::remove | ( | const std::string & | _name | ) |
Remove Value with his name.
- Parameters
-
[in] _name Name of the object
§ remove() [2/2]
void ejson::internal::Object::remove | ( | size_t | _id | ) |
Remove Value with his id.
- Parameters
-
[in] _id Id of the element.
§ size()
size_t ejson::internal::Object::size | ( | ) | const |
get the number of sub element in the current one
- Returns
- the Number of stored element
§ transfertIn()
|
overridevirtual |
Tranfert all element in the element set in parameter.
- Parameters
-
[in,out] _obj move all parameter in the selected element
- Returns
- true if transfer is done corectly
- Note
- all element is remove from the curent element.
Reimplemented from ejson::internal::Value.
Member Data Documentation
§ m_value
|
protected |
value of the node (for element this is the name, for text it is the inside text ...)
The documentation for this class was generated from the following file:
- framework/atria-soft/ejson/ejson/internal/Object.hpp