#include <Node.hpp>
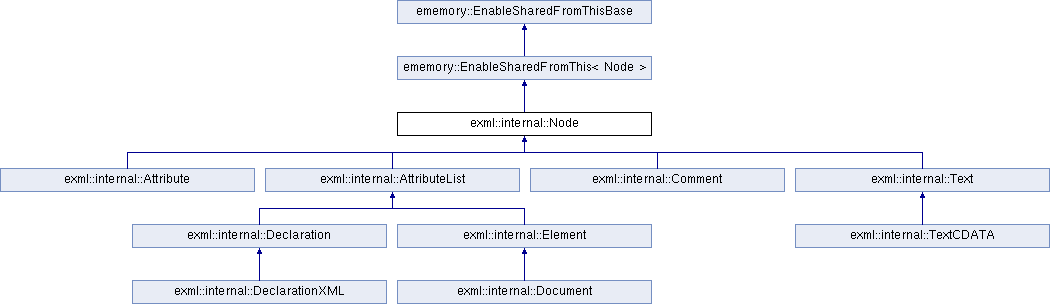
Protected Member Functions | |
Node () | |
Node (const std::string &_value) | |
void | addIndent (std::string &_data, int32_t _indent) const |
void | drawElementParsed (char32_t _val, const exml::FilePos &_filePos) const |
bool | checkAvaillable (char32_t _val, bool _firstChar) const |
int32_t | countWhiteChar (const std::string &_data, int32_t _pos, exml::FilePos &_filePos) const |
Protected Attributes | |
exml::FilePos | m_pos |
std::string | m_value |
Detailed Description
Basic main object of all xml elements.
Constructor & Destructor Documentation
§ Node() [1/2]
|
inlineprotected |
basic element of a xml structure
§ Node() [2/2]
|
protected |
basic element of a xml structure
- Parameters
-
[in] _value value of the node
§ ~Node()
|
virtualdefault |
Virtualize destructor.
Member Function Documentation
§ addIndent()
|
protected |
add indentation of the string input.
- Parameters
-
[in,out] _data String where the indentation is done. [in] _indent Number of tab to add at the string.
§ checkAvaillable()
|
protected |
check if an element or attribute is availlable (not : !"#$%&'()*+,/;<=>?@[]^`{|}~ \n\t\r and for first char : not -.0123456789).
- Parameters
-
[in] _val Value to check the conformity. [in] _firstChar True if the element check is the first char.
- Returns
- true The value can be a part of attribute name
- false The value can NOT be a part of attribute name
§ clear()
|
virtual |
clear the Node
Reimplemented in exml::internal::Element, exml::internal::AttributeList, and exml::internal::Attribute.
§ countWhiteChar()
|
protected |
count the number of white char in the string from the specify position (stop at the first element that is not a white char)
- Parameters
-
[in] _data Data to parse. [in] _pos Start position in the string. [out] _filePos new poistion of te file to add.
- Returns
- number of white element.
§ drawElementParsed()
|
protected |
Display the cuurent element that is curently parse.
- Parameters
-
[in] _val Char that is parsed. [in] _filePos Position of the char in the file.
§ getPos()
const exml::FilePos& exml::internal::Node::getPos | ( | ) | const |
get the current position where the element is in the file
- Returns
- The file position reference
§ getType()
|
virtual |
get the node type.
- Returns
- the type of the Node.
Reimplemented in exml::internal::Element, exml::internal::Document, exml::internal::Attribute, exml::internal::Text, exml::internal::Declaration, and exml::internal::Comment.
§ getValue()
|
virtual |
get the current element Value.
- Returns
- the reference of the string value.
§ iGenerate()
|
virtual |
generate a string with the tree of the xml
- Parameters
-
[in,out] _data string where to add the elements [in] _indent current indentation of the file
- Returns
- false if an error occured.
Reimplemented in exml::internal::Element, exml::internal::Document, exml::internal::AttributeList, exml::internal::TextCDATA, exml::internal::Attribute, exml::internal::Text, exml::internal::Comment, and exml::internal::Declaration.
§ iParse()
|
pure virtual |
parse the Current node [pure VIRUAL]
- Parameters
-
[in] _data data string to parse. [in,out] _pos position in the string to start parse, return the position end of parsing. [in] _caseSensitive Request a parsion of element that is not case sensitive (all element is in low case) [in,out] _filePos file parsing position (line x col x) [in,out] _doc Base document reference
- Returns
- false if an error occured.
Implemented in exml::internal::Element, exml::internal::TextCDATA, exml::internal::Attribute, exml::internal::Text, exml::internal::Declaration, and exml::internal::Comment.
§ isAttribute()
bool exml::internal::Node::isAttribute | ( | ) | const |
check if the node is a exml::internal::Attribute
- Returns
- true if the node is a exml::internal::Attribute
§ isComment()
bool exml::internal::Node::isComment | ( | ) | const |
check if the node is a exml::internal::Comment
- Returns
- true if the node is a exml::internal::Comment
§ isDeclaration()
bool exml::internal::Node::isDeclaration | ( | ) | const |
check if the node is a exml::internal::Declaration
- Returns
- true if the node is a exml::internal::Declaration
§ isDocument()
bool exml::internal::Node::isDocument | ( | ) | const |
check if the node is a exml::internal::Document
- Returns
- true if the node is a exml::internal::Document
§ isElement()
bool exml::internal::Node::isElement | ( | ) | const |
check if the node is a exml::internal::Element
- Returns
- true if the node is a exml::internal::Element
§ isText()
bool exml::internal::Node::isText | ( | ) | const |
check if the node is a exml::internal::Text
- Returns
- true if the node is a exml::internal::Text
§ setValue()
|
virtual |
set the value of the node.
- Parameters
-
[in] _value New value of the node.
§ toAttribute() [1/2]
|
virtual |
Cast the element in a Attribute if it is possible.
- Returns
- pointer on the class or nullptr.
Reimplemented in exml::internal::Attribute.
§ toAttribute() [2/2]
|
virtual |
Cast the element in a Attribute if it is possible.
- Returns
- CONST pointer on the class or nullptr.
Reimplemented in exml::internal::Attribute.
§ toComment() [1/2]
|
virtual |
Cast the element in a Comment if it is possible.
- Returns
- pointer on the class or nullptr.
Reimplemented in exml::internal::Comment.
§ toComment() [2/2]
|
virtual |
Cast the element in a Comment if it is possible.
- Returns
- CONST pointer on the class or nullptr.
Reimplemented in exml::internal::Comment.
§ toDeclaration() [1/2]
|
virtual |
Cast the element in a Declaration if it is possible.
- Returns
- pointer on the class or nullptr.
Reimplemented in exml::internal::Declaration.
§ toDeclaration() [2/2]
|
virtual |
Cast the element in a Declaration if it is possible.
- Returns
- CONST pointer on the class or nullptr.
Reimplemented in exml::internal::Declaration.
§ toDocument() [1/2]
|
virtual |
Cast the element in a Document if it is possible.
- Returns
- pointer on the class or nullptr.
Reimplemented in exml::internal::Document.
§ toDocument() [2/2]
|
virtual |
Cast the element in a Document if it is possible.
- Returns
- CONST pointer on the class or nullptr.
Reimplemented in exml::internal::Document.
§ toElement() [1/2]
|
virtual |
Cast the element in a Element if it is possible.
- Returns
- pointer on the class or nullptr.
Reimplemented in exml::internal::Element.
§ toElement() [2/2]
|
virtual |
Cast the element in a Element if it is possible.
- Returns
- CONST pointer on the class or nullptr.
Reimplemented in exml::internal::Element.
§ toText() [1/2]
|
virtual |
Cast the element in a Text if it is possible.
- Returns
- pointer on the class or nullptr.
Reimplemented in exml::internal::Text.
§ toText() [2/2]
|
virtual |
Cast the element in a Text if it is possible.
- Returns
- CONST pointer on the class or nullptr.
Reimplemented in exml::internal::Text.
Member Data Documentation
§ m_pos
|
protected |
position in the readed file == > not correct when the file is generated
§ m_value
|
protected |
value of the node (for element this is the name, for text it is the inside text ...)
The documentation for this class was generated from the following file:
- framework/atria-soft/exml/exml/internal/Node.hpp