exml::internal::AttributeList Class Reference
#include <AttributeList.hpp>
Inheritance diagram for exml::internal::AttributeList:
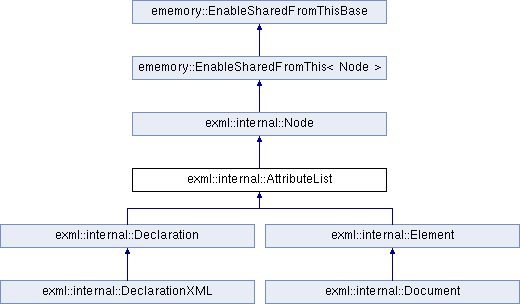
Public Member Functions | |
size_t | sizeAttribute () const |
void | appendAttribute (const ememory::SharedPtr< exml::internal::Attribute > &_attr) |
ememory::SharedPtr< Attribute > | getAttr (int32_t _id) |
ememory::SharedPtr< const Attribute > | getAttr (int32_t _id) const |
std::pair< std::string, std::string > | getAttrPair (int32_t _id) const |
const std::string & | getAttribute (const std::string &_name) const |
bool | existAttribute (const std::string &_name) const |
void | setAttribute (const std::string &_name, const std::string &_value) |
bool | removeAttribute (const std::string &_name) |
bool | iGenerate (std::string &_data, int32_t _indent) const override |
void | clear () override |
![]() | |
virtual | ~Node ()=default |
virtual bool | iParse (const std::string &_data, int32_t &_pos, bool _caseSensitive, exml::FilePos &_filePos, exml::internal::Document &_doc)=0 |
const exml::FilePos & | getPos () const |
virtual void | setValue (std::string _value) |
virtual const std::string & | getValue () const |
virtual enum nodeType | getType () const |
virtual ememory::SharedPtr< exml::internal::Document > | toDocument () |
virtual const ememory::SharedPtr< exml::internal::Document > | toDocument () const |
virtual ememory::SharedPtr< exml::internal::Attribute > | toAttribute () |
virtual const ememory::SharedPtr< exml::internal::Attribute > | toAttribute () const |
virtual ememory::SharedPtr< exml::internal::Comment > | toComment () |
virtual const ememory::SharedPtr< exml::internal::Comment > | toComment () const |
virtual ememory::SharedPtr< exml::internal::Declaration > | toDeclaration () |
virtual const ememory::SharedPtr< exml::internal::Declaration > | toDeclaration () const |
virtual ememory::SharedPtr< exml::internal::Element > | toElement () |
virtual const ememory::SharedPtr< exml::internal::Element > | toElement () const |
virtual ememory::SharedPtr< exml::internal::Text > | toText () |
virtual const ememory::SharedPtr< exml::internal::Text > | toText () const |
bool | isDocument () const |
bool | isAttribute () const |
bool | isComment () const |
bool | isDeclaration () const |
bool | isElement () const |
bool | isText () const |
![]() | |
ememory::SharedPtr< EMEMORY_TYPE > | sharedFromThis () |
const ememory::SharedPtr< EMEMORY_TYPE > | sharedFromThis () const |
ememory::WeakPtr< EMEMORY_TYPE > | weakFromThis () |
const ememory::WeakPtr< EMEMORY_TYPE > | weakFromThis () const |
Protected Member Functions | |
AttributeList (const std::string &_value="") | |
![]() | |
Node () | |
Node (const std::string &_value) | |
void | addIndent (std::string &_data, int32_t _indent) const |
void | drawElementParsed (char32_t _val, const exml::FilePos &_filePos) const |
bool | checkAvaillable (char32_t _val, bool _firstChar) const |
int32_t | countWhiteChar (const std::string &_data, int32_t _pos, exml::FilePos &_filePos) const |
Protected Attributes | |
std::vector< ememory::SharedPtr< exml::internal::Attribute > > | m_listAttribute |
![]() | |
exml::FilePos | m_pos |
std::string | m_value |
Detailed Description
List of all attribute element in a node.
Constructor & Destructor Documentation
§ AttributeList()
|
inlineprotected |
Constructor.
- Parameters
-
[in] _value Node value;
Member Function Documentation
§ appendAttribute()
void exml::internal::AttributeList::appendAttribute | ( | const ememory::SharedPtr< exml::internal::Attribute > & | _attr | ) |
add attribute on the List
- Parameters
-
[in] _attr Pointer on the attribute
§ clear()
|
overridevirtual |
§ existAttribute()
bool exml::internal::AttributeList::existAttribute | ( | const std::string & | _name | ) | const |
check if an attribute exist or not with his name.
- Parameters
-
[in] _name Attribute Name.
- Returns
- true if the attribute exist or False
§ getAttr() [1/2]
ememory::SharedPtr<Attribute> exml::internal::AttributeList::getAttr | ( | int32_t | _id | ) |
get attribute whith his ID
- Parameters
-
[in] _id Identifier of the attribute 0<= _id < sizeAttribute()
- Returns
- Pointer on the attribute or NULL
§ getAttr() [2/2]
ememory::SharedPtr<const Attribute> exml::internal::AttributeList::getAttr | ( | int32_t | _id | ) | const |
get attribute whith his ID
- Parameters
-
[in] _id Identifier of the attribute 0<= _id < sizeAttribute()
- Returns
- Pointer on the attribute or NULL
§ getAttribute()
const std::string& exml::internal::AttributeList::getAttribute | ( | const std::string & | _name | ) | const |
get the attribute value with searching in the List with his name
- Parameters
-
[in] _name Attribute Name.
- Returns
- Value of the attribute or no data in the string
§ getAttrPair()
std::pair<std::string, std::string> exml::internal::AttributeList::getAttrPair | ( | int32_t | _id | ) | const |
get attribute whith his ID
- Parameters
-
[in] _id Identifier of the attribute 0<= _id < sizeAttribute()
- Returns
- Name and value of the attribute
§ iGenerate()
|
overridevirtual |
generate a string with the tree of the xml
- Parameters
-
[in,out] _data string where to add the elements [in] _indent current indentation of the file
- Returns
- false if an error occured.
Reimplemented from exml::internal::Node.
Reimplemented in exml::internal::Element, exml::internal::Document, and exml::internal::Declaration.
§ removeAttribute()
bool exml::internal::AttributeList::removeAttribute | ( | const std::string & | _name | ) |
Remove an attribute form the list.
- Parameters
-
[in] _name Name of the attribute
- Returns
- true The attribute has been removed
- false An error occured.
§ setAttribute()
void exml::internal::AttributeList::setAttribute | ( | const std::string & | _name, |
const std::string & | _value | ||
) |
Set A new attribute or replace data of the previous one.
- Parameters
-
[in] _name Name of the attribute [in] _value Value of the attribute
§ sizeAttribute()
|
inline |
get the number of attribute in the Node
- Returns
- Nulber of attribute >=0
Member Data Documentation
§ m_listAttribute
|
protected |
list of all attribute
The documentation for this class was generated from the following file:
- framework/atria-soft/exml/exml/internal/AttributeList.hpp