#include <Node.hpp>
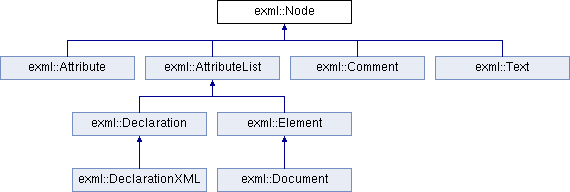
Public Member Functions | |
Node (const ememory::SharedPtr< exml::internal::Node > &_internalNode) | |
Node () | |
virtual | ~Node ()=default |
bool | exist () const |
exml::FilePos | getPos () const |
virtual void | setValue (std::string _value) |
const std::string & | getValue () const |
enum nodeType | getType () const |
exml::Document | toDocument () |
const exml::Document | toDocument () const |
exml::Attribute | toAttribute () |
const exml::Attribute | toAttribute () const |
exml::Comment | toComment () |
const exml::Comment | toComment () const |
exml::Declaration | toDeclaration () |
const exml::Declaration | toDeclaration () const |
exml::Element | toElement () |
const exml::Element | toElement () const |
exml::Text | toText () |
const exml::Text | toText () const |
bool | isDocument () const |
bool | isAttribute () const |
bool | isComment () const |
bool | isDeclaration () const |
bool | isElement () const |
bool | isText () const |
virtual void | clear () |
Protected Attributes | |
ememory::SharedPtr< exml::internal::Node > | m_data |
Friends | |
class | exml::Element |
class | exml::AttributeListData |
class | exml::ElementData |
Detailed Description
Basic main object of all xml elements.
Constructor & Destructor Documentation
§ Node() [1/2]
exml::Node::Node | ( | const ememory::SharedPtr< exml::internal::Node > & | _internalNode | ) |
basic element of a xml structure
- Parameters
-
[in] _internalNode Internal reference of the Node
§ Node() [2/2]
exml::Node::Node | ( | ) |
basic element of a xml structure
§ ~Node()
|
virtualdefault |
Virtualize destructor.
Member Function Documentation
§ clear()
|
virtual |
clear the Node
Reimplemented in exml::Element, and exml::Attribute.
§ exist()
bool exml::Node::exist | ( | ) | const |
Check if the element exit.
- Returns
- true The element exist
- false The element does NOT exist
§ getPos()
exml::FilePos exml::Node::getPos | ( | ) | const |
get the current position where the element is in the file
- Returns
- The file position reference
§ getType()
§ getValue()
const std::string& exml::Node::getValue | ( | ) | const |
get the current element Value.
- Returns
- the reference of the string value.
§ isAttribute()
bool exml::Node::isAttribute | ( | ) | const |
check if the node is a exml::Attribute
- Returns
- true if the node is a exml::Attribute
§ isComment()
bool exml::Node::isComment | ( | ) | const |
check if the node is a exml::Comment
- Returns
- true if the node is a exml::Comment
§ isDeclaration()
bool exml::Node::isDeclaration | ( | ) | const |
check if the node is a exml::Declaration
- Returns
- true if the node is a exml::Declaration
§ isDocument()
bool exml::Node::isDocument | ( | ) | const |
check if the node is a exml::Document
- Returns
- true if the node is a exml::Document
§ isElement()
bool exml::Node::isElement | ( | ) | const |
check if the node is a exml::Element
- Returns
- true if the node is a exml::Element
§ isText()
bool exml::Node::isText | ( | ) | const |
check if the node is a exml::Text
- Returns
- true if the node is a exml::Text
§ setValue()
|
virtual |
set the value of the node.
- Parameters
-
[in] _value New value of the node.
§ toAttribute() [1/2]
exml::Attribute exml::Node::toAttribute | ( | ) |
Cast the element in a Attribute if it is possible.
- Returns
- pointer on the class or nullptr.
§ toAttribute() [2/2]
const exml::Attribute exml::Node::toAttribute | ( | ) | const |
Cast the element in a Attribute if it is possible.
- Returns
- CONST pointer on the class or nullptr.
§ toComment() [1/2]
exml::Comment exml::Node::toComment | ( | ) |
Cast the element in a Comment if it is possible.
- Returns
- pointer on the class or nullptr.
§ toComment() [2/2]
const exml::Comment exml::Node::toComment | ( | ) | const |
Cast the element in a Comment if it is possible.
- Returns
- CONST pointer on the class or nullptr.
§ toDeclaration() [1/2]
exml::Declaration exml::Node::toDeclaration | ( | ) |
Cast the element in a Declaration if it is possible.
- Returns
- pointer on the class or nullptr.
§ toDeclaration() [2/2]
const exml::Declaration exml::Node::toDeclaration | ( | ) | const |
Cast the element in a Declaration if it is possible.
- Returns
- CONST pointer on the class or nullptr.
§ toDocument() [1/2]
exml::Document exml::Node::toDocument | ( | ) |
Cast the element in a Document if it is possible.
- Returns
- pointer on the class or nullptr.
§ toDocument() [2/2]
const exml::Document exml::Node::toDocument | ( | ) | const |
Cast the element in a Document if it is possible.
- Returns
- CONST pointer on the class or nullptr.
§ toElement() [1/2]
exml::Element exml::Node::toElement | ( | ) |
Cast the element in a Element if it is possible.
- Returns
- pointer on the class or nullptr.
§ toElement() [2/2]
const exml::Element exml::Node::toElement | ( | ) | const |
Cast the element in a Element if it is possible.
- Returns
- CONST pointer on the class or nullptr.
§ toText() [1/2]
exml::Text exml::Node::toText | ( | ) |
Cast the element in a Text if it is possible.
- Returns
- pointer on the class or nullptr.
§ toText() [2/2]
const exml::Text exml::Node::toText | ( | ) | const |
Cast the element in a Text if it is possible.
- Returns
- CONST pointer on the class or nullptr.
Member Data Documentation
§ m_data
|
protected |
internal reference on a node
The documentation for this class was generated from the following file:
- framework/atria-soft/exml/exml/Node.hpp