#include <Array.hpp>
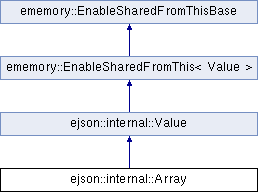
Public Member Functions | |
size_t | size () const |
ememory::SharedPtr< ejson::internal::Value > | get (size_t _id) |
const ememory::SharedPtr< ejson::internal::Value > | get (size_t _id) const |
bool | add (ememory::SharedPtr< ejson::internal::Value > _element) |
void | remove (size_t _id) |
bool | iParse (const std::string &_data, size_t &_pos, ejson::FilePos &_filePos, ejson::internal::Document &_doc) override |
bool | iGenerate (std::string &_data, size_t _indent) const override |
void | iMachineGenerate (std::string &_data) const override |
void | clear () override |
bool | transfertIn (ememory::SharedPtr< ejson::internal::Value > _obj) override |
ememory::SharedPtr< ejson::internal::Value > | clone () const override |
![]() | |
enum ejson::valueType | getType () const |
virtual | ~Value () |
void | display () const |
![]() | |
ememory::SharedPtr< EMEMORY_TYPE > | sharedFromThis () |
const ememory::SharedPtr< EMEMORY_TYPE > | sharedFromThis () const |
ememory::WeakPtr< EMEMORY_TYPE > | weakFromThis () |
const ememory::WeakPtr< EMEMORY_TYPE > | weakFromThis () const |
Static Public Member Functions | |
static ememory::SharedPtr< Array > | create () |
Protected Member Functions | |
Array () | |
![]() | |
Value () | |
void | addIndent (std::string &_data, int32_t _indent) const |
void | drawElementParsed (char32_t _val, const ejson::FilePos &_filePos) const |
bool | checkString (char32_t _val) const |
bool | checkNumber (char32_t _val) const |
int32_t | countWhiteChar (const std::string &_data, size_t _pos, ejson::FilePos &_filePos) const |
Additional Inherited Members | |
![]() | |
static bool | isWhiteChar (char32_t _val) |
![]() | |
enum ejson::valueType | m_type |
Detailed Description
ejson Array internal data implementation.
Constructor & Destructor Documentation
§ Array()
|
inlineprotected |
basic element of a xml structure
Member Function Documentation
§ add()
bool ejson::internal::Array::add | ( | ememory::SharedPtr< ejson::internal::Value > | _element | ) |
add an element on the array.
- Parameters
-
[in] _element element to add.
- Returns
- false if an error occured.
§ clear()
|
overridevirtual |
clear the Node
Reimplemented from ejson::internal::Value.
§ clone()
|
overridevirtual |
Copy the curent node and all the child in the curent one.
- Returns
- nullptr in an error occured, the pointer on the element otherwise
Reimplemented from ejson::internal::Value.
§ create()
|
static |
Create factory on the ejson::internal::Array.
- Returns
- A SharedPtr on the Array value
§ get() [1/2]
ememory::SharedPtr<ejson::internal::Value> ejson::internal::Array::get | ( | size_t | _id | ) |
get the pointer on an element reference with his ID.
- Parameters
-
[in] _id Id of the element.
- Returns
- nullptr if the element does not exist.
§ get() [2/2]
const ememory::SharedPtr<ejson::internal::Value> ejson::internal::Array::get | ( | size_t | _id | ) | const |
get the const pointer on an element reference with his ID.
- Parameters
-
[in] _id Id of the element.
- Returns
- nullptr if the element does not exist.
§ iGenerate()
|
overridevirtual |
generate a string with the tree of the json
- Parameters
-
[in,out] _data string where to add the elements [in] _indent current indentation of the file
- Returns
- false if an error occured.
Implements ejson::internal::Value.
§ iMachineGenerate()
|
overridevirtual |
generate a string with the tree of the json (not human readable ==> for computer transfer)
- Parameters
-
[in,out] _data string where to add the elements
- Returns
- false if an error occured.
Implements ejson::internal::Value.
§ iParse()
|
overridevirtual |
parse the Current node [pure VIRUAL]
- Parameters
-
[in] _data data string to parse. [in,out] _pos position in the string to start parse, return the position end of parsing. [in,out] _filePos Position in the file (in X/Y) [in,out] _doc Reference on the main document
- Returns
- false if an error occured.
Implements ejson::internal::Value.
§ remove()
void ejson::internal::Array::remove | ( | size_t | _id | ) |
Remove Value with his Id.
- Parameters
-
[in] _id Id of the element.
§ size()
size_t ejson::internal::Array::size | ( | ) | const |
get the number of sub element in the current one
- Returns
- the Number of stored element
§ transfertIn()
|
overridevirtual |
Tranfert all element in the element set in parameter.
- Parameters
-
[in,out] _obj move all parameter in the selected element
- Returns
- true if transfer is done corectly
- Note
- all element is remove from the curent element.
Reimplemented from ejson::internal::Value.
The documentation for this class was generated from the following file:
- framework/atria-soft/ejson/ejson/internal/Array.hpp