ejson::Object Class Reference
#include <Object.hpp>
Inheritance diagram for ejson::Object:
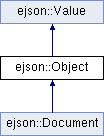
Public Types | |
using | iterator = ejson::iterator< ejson::Object > |
Additional Inherited Members | |
![]() | |
ememory::SharedPtr< ejson::internal::Value > | m_data |
Detailed Description
ejson Object interface { ... }.
Member Typedef Documentation
§ iterator
Specify iterator of the Object methode.
Constructor & Destructor Documentation
§ Object() [1/4]
ejson::Object::Object | ( | ememory::SharedPtr< ejson::internal::Value > | _internalValue | ) |
Constructor.
- Parameters
-
[in] _internalValue Internal Value to set data
§ Object() [2/4]
ejson::Object::Object | ( | const ejson::Object & | _obj | ) |
Copy constructor.
- Parameters
-
[in] _obj Object to copy
§ Object() [3/4]
ejson::Object::Object | ( | ) |
Constructor.
§ Object() [4/4]
ejson::Object::Object | ( | const std::string & | _data | ) |
Constructor.
- Parameters
-
[in] _data string data to parse
Member Function Documentation
§ add()
bool ejson::Object::add | ( | const std::string & | _name, |
const ejson::Value & | _value | ||
) |
add an element in the Object
- Parameters
-
[in] _name name of the object [in] _value Element to add
- Returns
- false if an error occured
§ begin() [1/2]
iterator ejson::Object::begin | ( | ) |
§ begin() [2/2]
const iterator ejson::Object::begin | ( | ) | const |
§ end() [1/2]
iterator ejson::Object::end | ( | ) |
§ end() [2/2]
const iterator ejson::Object::end | ( | ) | const |
§ getKey()
std::string ejson::Object::getKey | ( | size_t | _id | ) | const |
Get the element name (key).
- Parameters
-
[in] _id Id of the element.
- Returns
- The name (key).
§ getKeys()
std::vector<std::string> ejson::Object::getKeys | ( | ) | const |
Get all the element name (keys).
- Returns
- a vector of all name (key).
§ operator=()
ejson::Object& ejson::Object::operator= | ( | const ejson::Object & | _obj | ) |
§ operator[]() [1/4]
ejson::Value ejson::Object::operator[] | ( | const std::string & | _name | ) |
Cet the sub element with his name (no cast check)
- Parameters
-
[in] _name Name of the object
- Returns
- Value on the element requested or a value that does not exist ejson::Value::exist.
§ operator[]() [2/4]
const ejson::Value ejson::Object::operator[] | ( | const std::string & | _name | ) | const |
Get the const sub element with his name (no cast check)
- Parameters
-
[in] _name Name of the object
- Returns
- const Value on the element requested or a value that does not exist ejson::Value::exist.
§ operator[]() [3/4]
ejson::Value ejson::Object::operator[] | ( | size_t | _id | ) |
Get the value on an element reference with his ID.
- Parameters
-
[in] _id Id of the element.
- Returns
- Value on the element requested or a value that does not exist ejson::Value::exist.
§ operator[]() [4/4]
const ejson::Value ejson::Object::operator[] | ( | size_t | _id | ) | const |
Get the const value on an element reference with his ID.
- Parameters
-
[in] _id Id of the element.
- Returns
- const Value on the element requested or a value that does not exist ejson::Value::exist.
§ remove() [1/3]
void ejson::Object::remove | ( | const std::string & | _name | ) |
Remove Value with his name.
- Parameters
-
[in] _name Name of the object
§ remove() [2/3]
void ejson::Object::remove | ( | size_t | _id | ) |
Remove Value with his id.
- Parameters
-
[in] _id Id of the element.
§ remove() [3/3]
§ size()
size_t ejson::Object::size | ( | ) | const |
get the number of sub element in the current one
- Returns
- the Number of stored element
§ valueExist()
bool ejson::Object::valueExist | ( | const std::string & | _name | ) | const |
check if an element exist.
- Parameters
-
[in] _name Name of the object.
- Returns
- The existance of the element.
The documentation for this class was generated from the following file:
- framework/atria-soft/ejson/ejson/Object.hpp