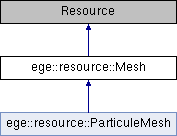
Public Types | |
enum | normalMode { normalModeNone, normalModeFace, normalModeVertex } |
Public Member Functions | |
DECLARE_RESOURCE_NAMED_FACTORY (Mesh) | |
virtual void | draw (mat4 &_positionMatrix, bool _enableDepthTest=true, bool _enableDepthUpdate=true) |
virtual void | draw (mat4 &_positionMatrix, const etk::Color< float > &_mainColor, bool _enableDepthTest=true, bool _enableDepthUpdate=true) |
void | generateVBO () |
void | createViewBox (const std::string &_materialName, float _size=1.0) |
void | createIcoSphere (const std::string &_materialName, float _size=1.0, int32_t _subdivision=3) |
void | addMaterial (const std::string &_name, ememory::SharedPtr< ege::Material > _data) |
void | setCheckNormal (bool _status) |
bool | getCheckNormal () |
const std::vector< ememory::SharedPtr< ege::PhysicsShape > > & | getPhysicalProperties () const |
void | addPhysicElement (const ememory::SharedPtr< ege::PhysicsShape > &_shape) |
void | setShape (void *_shape) |
void * | getShape () |
void | setFreeShapeFunction (void(*_functionFreeShape)(void *_pointer)) |
void | addFaceIndexing (const std::string &_layerName) |
void | addPoint (const std::string &_layerName, const vec3 &_pos, const etk::Color< float > &_color) |
void | addLine (const std::string &_layerName, const vec3 &_pos1, const vec3 &_pos2, const etk::Color< float > &_color) |
void | addLine (const std::string &_layerName, const vec3 &_pos1, const vec3 &_pos2, const etk::Color< float > &_color1, const etk::Color< float > &_color2) |
void | addLines (const std::string &_layerName, const std::vector< vec3 > &_list, const etk::Color< float > &_color) |
void | addLines (const std::string &_layerName, const std::vector< vec3 > &_list, const std::vector< etk::Color< float >> &_color) |
void | addTriangle (const std::string &_layerName, const vec3 &_pos1, const vec3 &_pos2, const vec3 &_pos3, const etk::Color< float > &_color) |
void | addTriangle (const std::string &_layerName, const vec3 &_pos1, const vec3 &_pos2, const vec3 &_pos3, const etk::Color< float > &_color1, const etk::Color< float > &_color2, const etk::Color< float > &_color3) |
void | addQuad (const std::string &_layerName, const vec3 &_pos1, const vec3 &_pos2, const vec3 &_pos3, const vec3 &_pos4, const etk::Color< float > &_color) |
void | addQuad (const std::string &_layerName, const vec3 &_pos1, const vec3 &_pos2, const vec3 &_pos3, const vec3 &_pos4, const etk::Color< float > &_color1, const etk::Color< float > &_color2, const etk::Color< float > &_color3, const etk::Color< float > &_color4) |
void | addTriangle (const std::string &_layerName, const vec3 &_pos1, const vec3 &_pos2, const vec3 &_pos3, const vec2 &_uv1, const vec2 &_uv2, const vec2 &_uv3, const etk::Color< float > &_color) |
void | addTriangle (const std::string &_layerName, const vec3 &_pos1, const vec3 &_pos2, const vec3 &_pos3, const vec2 &_uv1, const vec2 &_uv2, const vec2 &_uv3, const etk::Color< float > &_color1=etk::color::white, const etk::Color< float > &_color2=etk::color::white, const etk::Color< float > &_color3=etk::color::white) |
void | addQuad (const std::string &_layerName, const vec3 &_pos1, const vec3 &_pos2, const vec3 &_pos3, const vec3 &_pos4, const vec2 &_uv1, const vec2 &_uv2, const vec2 &_uv3, const vec2 &_uv4, const etk::Color< float > &_color) |
void | addQuad (const std::string &_layerName, const vec3 &_pos1, const vec3 &_pos2, const vec3 &_pos3, const vec3 &_pos4, const vec2 &_uv1, const vec2 &_uv2, const vec2 &_uv3, const vec2 &_uv4, const etk::Color< float > &_color1=etk::color::white, const etk::Color< float > &_color2=etk::color::white, const etk::Color< float > &_color3=etk::color::white, const etk::Color< float > &_color4=etk::color::white) |
Static Public Member Functions | |
static ememory::SharedPtr< ege::resource::Mesh > | createGrid (int32_t _lineCount, const vec3 &_position=vec3(0, 0, 0), float _size=1.0f, const std::string &_materialName="basics") |
static ememory::SharedPtr< ege::resource::Mesh > | createCube (float _size=1.0f, const std::string &_materialName="basics", const etk::Color< float > &_color=etk::color::white) |
Protected Member Functions | |
void | clean () |
void | init (const std::string &_fileName="---", const std::string &_shaderName="DATA:textured3D2.prog") |
int32_t | findPositionInList (const vec3 &_pos) |
int32_t | findTextureInList (const vec2 &_uv) |
int32_t | findColorInList (const etk::Color< float > &_color) |
Protected Attributes | |
enum normalMode | m_normalMode |
bool | m_checkNormal |
ememory::SharedPtr< gale::resource::Program > | m_GLprogram |
int32_t | m_GLPosition |
int32_t | m_GLMatrix |
int32_t | m_GLMatrixPosition |
int32_t | m_GLNormal |
int32_t | m_GLtexture |
int32_t | m_GLColor |
int32_t | m_bufferOfset |
int32_t | m_numberOfElments |
MaterialGlId | m_GLMaterial |
ege::Light | m_light |
std::vector< vec3 > | m_listVertex |
std::vector< vec2 > | m_listUV |
std::vector< etk::Color< float > > | m_listColor |
std::vector< vec3 > | m_listFacesNormal |
std::vector< vec3 > | m_listVertexNormal |
etk::Hash< FaceIndexing > | m_listFaces |
etk::Hash< ememory::SharedPtr< ege::Material > > | m_materials |
std::vector< ememory::SharedPtr< ege::PhysicsShape > > | m_physics |
ememory::SharedPtr< gale::resource::VirtualBufferObject > | m_verticesVBO |
Member Enumeration Documentation
§ normalMode
Member Function Documentation
§ addFaceIndexing()
void ege::resource::Mesh::addFaceIndexing | ( | const std::string & | _layerName | ) |
Add in the faces list the layer requested.
- Parameters
-
[in] _layerName face index to add
§ addQuad() [1/4]
|
inline |
draw a colored quad (usefull for debug and test)
- Parameters
-
[in] _layerName Material and face indexing layer name [in] _pos1 First point position [in] _pos2 Second point position [in] _pos3 Third point position [in] _pos4 faurth point position [in] _color color of all elements
§ addQuad() [2/4]
|
inline |
draw a colored quad (usefull for debug and test)
- Parameters
-
[in] _layerName Material and face indexing layer name [in] _pos1 First point position [in] _pos2 Second point position [in] _pos3 Third point position [in] _pos4 faurth point position [in] _color1 color of the _pos1 element [in] _color2 color of the _pos2 element [in] _color3 color of the _pos3 element [in] _color4 color of the _pos4 element
§ addQuad() [3/4]
|
inline |
draw a textured colored quad (usefull for debug and test)
- Parameters
-
[in] _layerName Material and face indexing layer name [in] _pos1 First point position [in] _pos2 Second point position [in] _pos3 Third point position [in] _pos4 faurth point position [in] _color color of all elements [in] _uv1 texture position of the _pos1 element [in] _uv2 texture position of the _pos2 element [in] _uv3 texture position of the _pos3 element [in] _uv4 texture position of the _pos4 element
§ addQuad() [4/4]
|
inline |
draw a textured quad (usefull for debug and test)
- Parameters
-
[in] _layerName Material and face indexing layer name [in] _pos1 First point position [in] _pos2 Second point position [in] _pos3 Third point position [in] _pos4 faurth point position [in] _uv1 texture position of the _pos1 element [in] _uv2 texture position of the _pos2 element [in] _uv3 texture position of the _pos3 element [in] _uv4 texture position of the _pos4 element [in] _color1 color of the _pos1 element [in] _color2 color of the _pos2 element [in] _color3 color of the _pos3 element [in] _color4 color of the _pos4 element
§ addTriangle() [1/4]
|
inline |
draw a colored triangle (usefull for debug and test)
- Parameters
-
[in] _layerName Material and face indexing layer name [in] _pos1 First point position [in] _pos2 Second point position [in] _pos3 Third point position [in] _color1 color of the _pos1 element [in] _color2 color of the _pos2 element [in] _color3 color of the _pos3 element
§ addTriangle() [2/4]
void ege::resource::Mesh::addTriangle | ( | const std::string & | _layerName, |
const vec3 & | _pos1, | ||
const vec3 & | _pos2, | ||
const vec3 & | _pos3, | ||
const etk::Color< float > & | _color1, | ||
const etk::Color< float > & | _color2, | ||
const etk::Color< float > & | _color3 | ||
) |
draw a colored triangle (usefull for debug and test)
- Parameters
-
[in] _layerName Material and face indexing layer name [in] _pos1 First point position [in] _pos2 Second point position [in] _pos3 Third point position [in] _color1 color of the _pos1 element [in] _color2 color of the _pos2 element [in] _color3 color of the _pos3 element
§ addTriangle() [3/4]
|
inline |
draw a textured colored triangle (usefull for debug and test)
- Parameters
-
[in] _layerName Material and face indexing layer name [in] _pos1 First point position [in] _pos2 Second point position [in] _pos3 Third point position [in] _color color of all elements [in] _uv1 texture position of the _pos1 element [in] _uv2 texture position of the _pos2 element [in] _uv3 texture position of the _pos3 element
§ addTriangle() [4/4]
void ege::resource::Mesh::addTriangle | ( | const std::string & | _layerName, |
const vec3 & | _pos1, | ||
const vec3 & | _pos2, | ||
const vec3 & | _pos3, | ||
const vec2 & | _uv1, | ||
const vec2 & | _uv2, | ||
const vec2 & | _uv3, | ||
const etk::Color< float > & | _color1 = etk::color::white , |
||
const etk::Color< float > & | _color2 = etk::color::white , |
||
const etk::Color< float > & | _color3 = etk::color::white |
||
) |
draw a textured colored triangle (usefull for debug and test)
- Parameters
-
[in] _layerName Material and face indexing layer name [in] _pos1 First point position [in] _pos2 Second point position [in] _pos3 Third point position [in] _color1 color of the _pos1 element [in] _color2 color of the _pos2 element [in] _color3 color of the _pos3 element [in] _uv1 texture position of the _pos1 element [in] _uv2 texture position of the _pos2 element [in] _uv3 texture position of the _pos3 element
§ getCheckNormal()
|
inline |
get the check value of normal position befor sending it to the openGl card
- Returns
- get the chcking stus of normal or not
§ getShape()
|
inline |
get the pointer on the shame (no type)
- Returns
- Pointer on shape.
§ setCheckNormal()
|
inline |
set the check of normal position befor sending it to the openGl card
- Parameters
-
[in] _status New state.
§ setShape()
void ege::resource::Mesh::setShape | ( | void * | _shape | ) |
set the shape pointer (no type == > user might know it ...)
- Parameters
-
[in] _shape The new shape (this remove the previous one)
Member Data Documentation
§ m_checkNormal
|
protected |
when enable, this check the normal of the mesh before sending it at the 3d card
§ m_listColor
|
protected |
List of all Color point in the mesh.
§ m_listFaces
|
protected |
List of all Face for the mesh.
§ m_listFacesNormal
|
protected |
List of all Face normal, when calculated.
§ m_listUV
|
protected |
List of all UV point in the mesh (for the specify texture)
§ m_listVertex
|
protected |
List of all vertex in the element.
§ m_listVertexNormal
|
protected |
List of all Face normal, when calculated.
§ m_physics
|
protected |
collision shape module ... (independent of bullet lib)
The documentation for this class was generated from the following file:
- framework/atria-soft/ege/ege/resource/Mesh.hpp