#include <Buffer.hpp>
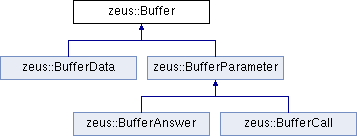
Public Types | |
enum | typeMessage { typeMessage::unknow = 0x0000, typeMessage::call = 0x0001, typeMessage::answer = 0x0002, typeMessage::data = 0x0003, typeMessage::event = 0x0004 } |
Public Member Functions | |
virtual | ~Buffer ()=default |
bool | haveAsync () const |
std::vector< zeus::ActionAsyncClient > | moveAsync () |
void | appendBuffer (ememory::SharedPtr< zeus::Buffer > _obj) |
virtual void | appendBufferData (ememory::SharedPtr< zeus::BufferData > _obj) |
void | clear () |
uint32_t | getTransactionId () const |
void | setTransactionId (uint32_t _value) |
uint32_t | getClientId () const |
void | setClientId (uint32_t _value) |
uint32_t | getServiceId () const |
void | setServiceId (uint32_t _value) |
bool | getPartFinish () const |
void | setPartFinish (bool _value) |
virtual enum typeMessage | getType () const |
virtual bool | writeOn (enet::WebSocket &_interface) |
virtual void | generateDisplay (std::ostream &_os) const |
Static Public Member Functions | |
static ememory::SharedPtr< zeus::Buffer > | create () |
static ememory::SharedPtr< zeus::Buffer > | create (const std::vector< uint8_t > &_buffer) |
Protected Member Functions | |
Buffer () | |
virtual void | composeWith (const uint8_t *_buffer, uint32_t _lenght) |
Protected Attributes | |
headerBin | m_header |
std::vector< zeus::ActionAsyncClient > | m_multipleSend |
Detailed Description
Protocol buffer to transmit datas.
Member Enumeration Documentation
§ typeMessage
|
strong |
Constructor & Destructor Documentation
§ Buffer()
|
protected |
basic constructor (hidden to force the use of ememory::SharedPtr) zeus::Buffer::create
§ ~Buffer()
|
virtualdefault |
Virtualize the buffer class
Member Function Documentation
§ appendBuffer()
void zeus::Buffer::appendBuffer | ( | ememory::SharedPtr< zeus::Buffer > | _obj | ) |
When multiple frame buffer, they need to concatenate the data... call this function with the new data to append it ...
- Parameters
-
[in] _obj Buffer to add
§ clear()
void zeus::Buffer::clear | ( | ) |
Chear the buffer.
§ composeWith()
|
protectedvirtual |
When receive new data form websocket, it might be added by this input (set all the frame ...)
- Parameters
-
[in] _buffer Pointer on the data to add. [in] _lenght number of octet to add.
Reimplemented in zeus::BufferParameter, zeus::BufferData, zeus::BufferAnswer, and zeus::BufferCall.
§ create() [1/2]
|
static |
Create a shared pointer on the buffer.
- Returns
- Allocated Buffer.
§ create() [2/2]
|
static |
§ getClientId()
uint32_t zeus::Buffer::getClientId | ( | ) | const |
§ getPartFinish()
bool zeus::Buffer::getPartFinish | ( | ) | const |
Check if it is the last packet of the buffer.
- Returns
- If "true" The Buffer wait no more datas
§ getServiceId()
|
inline |
§ getTransactionId()
uint32_t zeus::Buffer::getTransactionId | ( | ) | const |
Get the transaction identifier of the packet.
- Returns
- value of the transaction
§ getType()
|
virtual |
Get the type of the buffer.
- Returns
- the current type of the buffer
Reimplemented in zeus::BufferData, zeus::BufferAnswer, and zeus::BufferCall.
§ haveAsync()
|
inline |
Check if async element are present on this buffer.
- Returns
- return true if somme data must be send asyncronously
§ moveAsync()
|
inline |
Get the list of async data to send.
- Returns
- Vector of the async data (the async are moved out ... call only one time)
§ setClientId()
void zeus::Buffer::setClientId | ( | uint32_t | _value | ) |
§ setPartFinish()
void zeus::Buffer::setPartFinish | ( | bool | _value | ) |
set the finish state of the buffer
- Parameters
-
[in] _value set the sate of finish of the buffer
§ setServiceId()
|
inline |
§ setTransactionId()
void zeus::Buffer::setTransactionId | ( | uint32_t | _value | ) |
Set the transaction identifier of the packet.
- Parameters
-
[in] _value New transaction id
§ writeOn()
|
virtual |
Write the buffer on a specific interface.
- Parameters
-
[in] _interface socket to write data
- Returns
- true of no error appear
Reimplemented in zeus::BufferParameter, zeus::BufferData, zeus::BufferAnswer, and zeus::BufferCall.
Member Data Documentation
§ m_header
|
protected |
header of the protocol
§ m_multipleSend
|
protected |
Async element to send data on the webinterface when too big ...
The documentation for this class was generated from the following file:
- framework/atria-soft/zeus/zeus/Buffer.hpp